c# code for select all checkbox in wpf datagrid
Solution 1
This can be done declaratively. The following creates a checkbox column for each row and which can toggle row selections. The header of the checkbox column can be clicked to do a select all of the rows.
Relevant portions from the xaml
<Window x:Class="Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:toolkit="http://schemas.microsoft.com/wpf/2008/toolkit">
<toolkit:DataGrid Name="dataGrid"
ItemsSource="{Binding}" AutoGenerateColumns="True"
SelectionMode="Extended" CanResizeRows="False">
<toolkit:DataGrid.RowHeaderTemplate>
<DataTemplate>
<Grid>
<CheckBox IsChecked="{
Binding Path=IsSelected,
Mode=TwoWay,
RelativeSource={RelativeSource FindAncestor,
AncestorType={x:Type toolkit:DataGridRow}}}"
/>
</Grid>
</DataTemplate>
</toolkit:DataGrid.RowHeaderTemplate>
</toolkit:DataGrid>
</Window>
Solution 2
Here is the sample datagrid we use in the .Net 4.0 XAML file:
<DataGrid Grid.Row="0" Grid.Column="0" Grid.ColumnSpan="2" Name="dgMissingNames" ItemsSource="{Binding Path=TheMissingChildren}" Style="{StaticResource NameListGrid}" SelectionChanged="DataGrid_SelectionChanged">
<DataGrid.Columns>
<DataGridTemplateColumn CellStyle="{StaticResource NameListCol}">
<DataGridTemplateColumn.HeaderTemplate>
<DataTemplate>
<CheckBox Checked="CheckBox_Checked" Unchecked="CheckBox_Unchecked" />
</DataTemplate>
</DataGridTemplateColumn.HeaderTemplate>
<DataGridTemplateColumn.CellTemplate>
<DataTemplate>
<CheckBox IsChecked="{Binding Path=Checked, UpdateSourceTrigger=PropertyChanged}" Name="theCheckbox"/>
</DataTemplate>
</DataGridTemplateColumn.CellTemplate>
</DataGridTemplateColumn>
<DataGridTextColumn Binding="{Binding Path=SKU}" Header="Album" CellStyle="{StaticResource NameListCol}"/>
<DataGridTextColumn Binding="{Binding Path=Name}" Header="Name" CellStyle="{StaticResource NameListCol}"/>
<DataGridTextColumn Binding="{Binding Path=Pronunciation}" Header="Pronunciation" CellStyle="{StaticResource NameListCol}"/>
</DataGrid.Columns>
</DataGrid>
Here is the codebehind:
private void HeadCheck(object sender, RoutedEventArgs e, bool IsChecked)
{
foreach (CheckedMusicFile mf in TheMissingChildren)
{
mf.Checked = IsChecked;
}
dgMissingNames.Items.Refresh();
}
private void CheckBox_Checked(object sender, RoutedEventArgs e)
{
HeadCheck(sender, e, true);
}
private void CheckBox_Unchecked(object sender, RoutedEventArgs e)
{
HeadCheck(sender, e, false);
}
TheMissingChildren is a simple object structure with some string properties and an ischecked boolean.
HTH.
Solution 3
This is based on someone else's source that I can't recall, but we use it to help find visual children of a type. It may not be the most efficient use for this scenario but it might help get you on the right track.
public static childItem FindVisualChild<childItem>(DependencyObject obj) where childItem : DependencyObject
{
for (int i = 0; i < VisualTreeHelper.GetChildrenCount(obj); i++)
{
DependencyObject child = VisualTreeHelper.GetChild(obj, i);
if (child != null && child is childItem)
return (childItem)child;
childItem childOfChild = FindVisualChild<childItem>(child);
if (childOfChild != null)
return childOfChild;
}
return null;
}
[Edit 4.16.09] Based on that, try out this method. Should find all CheckBoxes and change the state as provided, callable from your event handler on the Checked/Unchecked events.
public static void CheckAllBoxes(DependencyObject obj, bool isChecked)
{
for (int i = 0; i < VisualTreeHelper.GetChildrenCount(obj); i++)
{
// If a checkbox, change IsChecked and continue.
if (obj is CheckBox)
{
((CheckBox) obj).IsChecked = isChecked;
continue;
}
DependencyObject child = VisualTreeHelper.GetChild(obj, i);
CheckAllBoxes(child, isChecked);
}
}
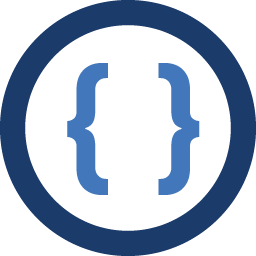
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I need some c# code to select / deselect all checkboxes in a datagrid in WPF 3.5 framework. I would like to do this by clicking a single header checkbox in the grid.
Please help.
-
Admin almost 13 yearsif you are not going to use the sender object or the routedeventargs in HeadCheck you shouldnt be passing them in
-
AechoLiu about 11 yearsThe
UpdateSourceTrigger=PropertyChanged
does help me. Thanks.