c# datetime format
Solution 1
I think this will do what you want:
System.Globalization.DateTimeFormatInfo format =
System.Globalization.CultureInfo.CurrentCulture.DateTimeFormat;
string dateTime = DateTime.Now.ToString(format.FullDateTimePattern);
EDIT:
You can do the following for a short date/time:
System.Globalization.DateTimeFormatInfo format =
System.Globalization.CultureInfo.CurrentCulture.DateTimeFormat;
string dateTime = DateTime.Now.ToString(format.ShortDatePattern + " " +
format.ShortTimePattern);
Solution 2
Instead of using "g", you might find the format you want in the Standard Date and Time Format Strings documentation page.
Edit: It looks like you may want CultureInfo.DateTimeFormat.ShortDatePattern, but check out the options anyway.
Solution 3
The /
character is a placeholder that will be replaced by the date separation character of the current culture. If you wish to "hard-code" the format to use /
you need to alter the format string like this:
dateTime.ToString("dd'/'MM'/'yyyy hh':'mm");
Solution 4
You do want to use "g", however the leading zero is dependant on the culture. The Invariant culture and some others will include the leading zero, but other cultures will not.
Any particular reason you want to maintain the leading zero?
Solution 5
try this:
var cinf = CultureInfo.CurrentCulture.DateTimeFormat.DateSeparator;
DateTime.Now.ToString("dd/MM/yyyy hh:mm").Replace(cinf,"/");
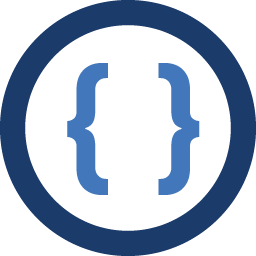
Admin
Updated on July 14, 2022Comments
-
Admin almost 2 years
I wish to have a specific format for my DateTime depending on the current culture.
So I try this:
dateTime.ToString("dd/MM/yyyy hh:mm");
This is partially OK, the / gets replaced by the culture-specific separator. But the day and month order are not switched (like MM/dd) depending on the culture.
Using
.ToString("g")
works, but that doesn't include the leading zero.How do I accomplish this?