C++ delete last character in a txt file
Solution 1
The only way to do this in portable code is to read in the data, and write out all but the last character.
If you don't mind non-portable code, most systems provide ways to truncate a file. The traditional Unix method is to seek to the place you want the file to end, and then do a write of 0 bytes to the file at that point. On Windows, you can use SetEndOfFile. Other systems will use different names and/or methods, but nearly all will have the capability in some form.
Solution 2
For a portable solution, something along these lines should do the job:
#include <fstream>
int main(){
std::ifstream fileIn( "file.txt" ); // Open for reading
std::string contents;
fileIn >> contents; // Store contents in a std::string
fileIn.close();
contents.pop_back(); // Remove last character
std::ofstream fileOut( "file.txt", std::ios::trunc ); // Open for writing (while also clearing file)
fileOut << contents; // Output contents with removed character
fileOut.close();
return 0;
}
Solution 3
Here's a more robust method, going off Alex Z's answer:
#include <fstream>
#include <string>
#include <sstream>
int main(){
std::ifstream fileIn( "file.txt" ); // Open for reading
std::stringstream buffer; // Store contents in a std::string
buffer << fileIn.rdbuf();
std::string contents = buffer.str();
fileIn.close();
contents.pop_back(); // Remove last character
std::ofstream fileOut( "file.txt" , std::ios::trunc); // Open for writing (while also clearing file)
fileOut << contents; // Output contents with removed character
fileOut.close();
}
The trick is these lines, which allow you to read the entire file efficiently into the string, and not just a token:
std::stringstream buffer;
buffer << fileIn.rdbuf();
std::string contents = buffer.str();
This is inspired from Jerry Coffin's first solution in this post. It is supposed to be the fastest solution there.
Solution 4
If the input file is not too large, you can do the following:-
1. Read the contents into a character array.
2. Truncate the original file.
3. Write the character array back to the file, except the last character.
If the file is too large, you can possibly use a temporary file instead of a character array. It will be a bit slow though.
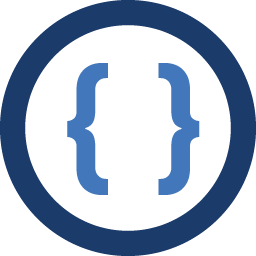
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I need some help on deleting the last character in a txt file. For example, if my txt file contains 1234567, I need the C++ code to delete the last character so that the file becomes 123456. Thanks guys.