C++ free all memory used by struct
You only need to delete
memory you allocate with new
.
printf would print 5.0, so the variable pointed to by a is not exactly destroyed.
You're actually running into undefined behavior. Although the value is still there, the memory was released and can be reused.
So the following:
struct CoordLocation
{
float X;
float Y;
float Z;
};
can't create a memory leak if you omit the destructor.
Your next snippet:
struct CoordLocation
{
float *X;
float *Y;
float *Z;
};
int main()
{
CoordLocation *coord = new CoordLocation();
delete coord;
return 0;
}
can potentially create a memory leak, but not as it is. The following will:
int main()
{
CoordLocation *coord = new CoordLocation();
coord->X = new float();
delete coord;
return 0;
}
Your third example
struct CoordLocation
{
CoordLocation()
{
*X = new float;
*Y = new float;
*Z = new float;
}
~CoordLocation()
{
delete X; delete Y; delete Z;
}
float *X;
float *Y;
float *Z;
};
int main()
{
CoordLocation *coord = new CoordLocation();
delete coord;
return 0;
}
won't create a memory leak because you free all the memory that you allocate. If you were to omit the destructor or forget to call delete coord;
, they you'd have a memory leak.
A good rule of thumb: call a delete
for every new
and a delete[]
for every new[]
and you're safe.
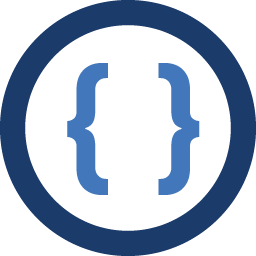
Admin
Updated on April 21, 2020Comments
-
Admin about 4 years
Quick question; I've googled around and found some answers already, but I'm a bit paranoid so I want to be sure.
Consider this situation:
struct CoordLocation { float X; float Y; float Z; }; int main() { CoordLocation *coord = new CoordLocation(); delete coord; return 0; }
Will calling delete also clear the memory used by the fields X, Y, Z? Some answers I found mentioned that I'd just delete the POINTER, not the actually referenced object this way. What if...
struct CoordLocation { float *X; float *Y; float *Z; }; int main() { CoordLocation *coord = new CoordLocation(); delete coord; return 0; }
And what if I manually free the memory for each object inside the struct's constructor/destructor?
struct CoordLocation { CoordLocation() { *X = new float; *Y = new float; *Z = new float; } ~CoordLocation() { delete X; delete Y; delete Z; } float *X; float *Y; float *Z; }; int main() { CoordLocation *coord = new CoordLocation(); delete coord; return 0; }
I noticed that for a simple situation such as:
float *a = new float; *a = 5.0f; printf("%f", *a); delete a; printf("%f", &a);
printf would print 5.0, so the variable pointed to by a is not exactly destroyed.
So my question is: How can I reliably free (as in no memory leaks) ALL the memory used by the struct in this case?
struct CoordLocation { float X; float Y; float Z; }; int main() { CoordLocation *coord = new CoordLocation(); delete coord; return 0; }
Thanks!
-
mjfgates about 12 yearsPerhaps, "WILL be reused." Said in a threatening tone of voice, while holding a lit flashlight under your chin.
-
Bojan Komazec about 12 yearsLuchian, what happens in third example if second or third
new
throws exception? There are no exception handlers inmain
soterminate
andabort
will be executed and program will be terminated but prior to that, isn't there a memory leak asdelete coord;
(and sodelete X;
) is never executed? -
Luchian Grigore about 12 years@BojanKomazec AH! It depends on what you mean by a memory leak - stackoverflow.com/questions/9921590/…
-
Mark B about 12 yearsThe third example will create a double delete if you ever copy construct one.
-
Bojan Komazec about 12 years@LuchianGrigore Yeah, that's one of those border cases. It might or might not be regarded as a leak; am still reading answers. You raised very interesting question there.
-
Piotr Dabkowski over 6 yearsAmazing answer! You should write tutorials ;)