C#: get information about computer in domain
12,903
Solution 1
WMI Library and here is a VB.net example. It shouldn't be difficult to convert it to C#
Solution 2
Checkout System.Management and System.Management.ManagementClass. Both are used for accessing WMI, which is how to get the information in question.
Edit: Updated with sample to access WMI from remote computer:
ConnectionOptions options;
options = new ConnectionOptions();
options.Username = userID;
options.Password = password;
options.EnablePrivileges = true;
options.Impersonation = ImpersonationLevel.Impersonate;
ManagementScope scope;
scope = new ManagementScope("\\\\" + ipAddress + "\\root\\cimv2", options);
scope.Connect();
String queryString = "SELECT PercentProcessorTime, PercentInterruptTime, InterruptsPersec FROM Win32_PerfFormattedData_PerfOS_Processor";
ObjectQuery query;
query = new ObjectQuery(queryString);
ManagementObjectSearcher objOS = new ManagementObjectSearcher(scope, query);
DataTable dt = new DataTable();
dt.Columns.Add("PercentProcessorTime");
dt.Columns.Add("PercentInterruptTime");
dt.Columns.Add("InterruptsPersec");
foreach (ManagementObject MO in objOS.Get())
{
DataRow dr = dt.NewRow();
dr["PercentProcessorTime"] = MO["PercentProcessorTime"];
dr["PercentInterruptTime"] = MO["PercentInterruptTime"];
dr["InterruptsPersec"] = MO["InterruptsPersec"];
dt.Rows.Add(dr);
}
Note: userID, password, and ipAddress must all be defined to match your environment.
Solution 3
Here is an example of using it in like an about box. MSDN has the rest of the items you can all.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Management;
namespace About_box
{
public partial class About : Form
{
public About()
{
InitializeComponent();
FormLoad();
}
public void FormLoad()
{
SystemInfo si;
SystemInfo.GetSystemInfo(out si);
txtboxApplication.Text = si.AppName;
txtboxVersion.Text = si.AppVersion;
txtBoxComputerName.Text = si.MachineName;
txtBoxMemory.Text = Convert.ToString((si.TotalRam / 1073741824)
+ " GigaBytes");
txtBoxProcessor.Text = si.ProcessorName;
txtBoxOperatingSystem.Text = si.OperatingSystem;
txtBoxOSVersion.Text = si.OperatingSystemVersion;
txtBoxManufacturer.Text = si.Manufacturer;
txtBoxModel.Text = si.Model;
}
}
}
Solution 4
Look into the WMI library.
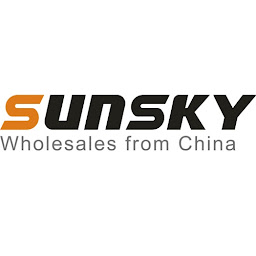
Author by
Sunskyonline
Updated on June 09, 2022Comments
-
Sunskyonline almost 2 years
What classes should I use in C# in order to get information about a certain computer in my network? (Like who is logged on that computer, what Operating System is running on that computer, what ports are opened etc)
-
Nate almost 15 yearsThis will only work if the code is run ON the machine in question; and will not work to get information about another machine on the network.