c# httpwebrequest getResponse() freezes and hangs my program
Solution 1
You're never closing the StreamWriter
... so I suspect it's not being flushed. Admittedly I'd expect an error from the server instead of just a hang, but it's worth looking at.
Btw, you don't need to close the response and dispose it. Just the using
statement is enough.
Solution 2
There's not much you can do if the remote server is not responding other than defining a Timeout
and catch the exception as you did in order to inform the user that the operation cannot complete because the remote site didn't respond:
var request = (HttpWebRequest)WebRequest.Create("https://qrua.com/qr/service/auth/login");
request.Timeout = 5000;
// If the server doesn't respond within 5 seconds you might catch the timeout exception
using (var response = request.GetResponse())
{
}
If you don't want to freeze the UI you could use the async version: BeginGetResponse
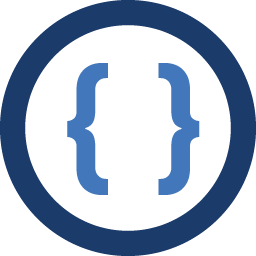
Admin
Updated on July 14, 2022Comments
-
Admin almost 2 years
I was trying to use httpwebrequest to use a rest like service on a remote server and from the first execution itself, my code was hanging the program. Then I tried it as a console application to make sure it has nothing to do with the program itself but no luck!
string credentialsJson = @"{""username"":""test"", ""password"":""test"" }"; int tmp = ServicePointManager.DefaultConnectionLimit; HttpWebRequest request = (HttpWebRequest)WebRequest.Create(@"https://qrua.com/qr/service" + @"/auth/login"); request.Method = "POST"; request.KeepAlive = true; request.Timeout = 50000 ; request.CookieContainer = new CookieContainer(); request.ContentType = "application/json"; try { StreamWriter writer = new StreamWriter(request.GetRequestStream()); writer.Write(credentialsJson); } catch (Exception e) { Console.WriteLine("EXCEPTION:" + e.Message); } //WebResponse response = request.GetResponse(); try { using (WebResponse response = (HttpWebResponse)request.GetResponse()) { Console.WriteLine("request:\n" + request.ToString() + "\nresponse:\n" + response.ContentLength); response.Close(); } } catch (Exception e) { Console.WriteLine("EXCEPTION: in sending http request:" + " \nError message:" + e.Message); }
Tried several things from different forums but it doesnt help. Even a simple console app with the above code hangs the console indefinitely! Any help would be great..
Thanks
-
Stephen Oberauer almost 13 yearsWhy did you mark this as correct, and then in another place you say, "tried closing the writer and it didnt do much" ?
-
Jon Skeet almost 13 years@Lost Hobbit: I suggest you add a comment to the question instead of to my answer - the OP won't get notification of this comment. (Not that the OP has been on Stack Overflow for 11 months anyway...)
-
Kenny Eliasson over 12 yearsWas using HtmlAgilityPack and following permanent redirects in that library didnt close the underlying response before creating a new.
-
jmrnet over 10 yearsThis exactly worked for me on the exact problem I was just having. Thanks @JonSkeet.
-
karliwson about 7 yearsHelped me a lot. Thanks.