C++: insert char to a string
Solution 1
There are a number of overloads of std::string::insert
. The overload for inserting a single character actually has three parameters:
string& insert(size_type pos, size_type n, char c);
The second parameter, n
, is the number of times to insert c
into the string at position pos
(i.e., the number of times to repeat the character. If you only want to insert one instance of the character, simply pass it one, e.g.,
someString.insert(somePosition, 1, myChar);
Solution 2
- Everything seems to be compiling successfully, but program crashes the gets to
conversion >> myCharInsert;
The problem is that you are trying to dereference(access) myCharInsert
(declared as a char*
) which is pointing to a random location in memory(which might not be inside the user's address space) and doing so is Undefined Behavior (crash on most implementations).
EDIT
To insert a char
into a string use string& insert ( size_t pos1, size_t n, char c );
overload.
Extra
To convert char
into a std::string
read this answer
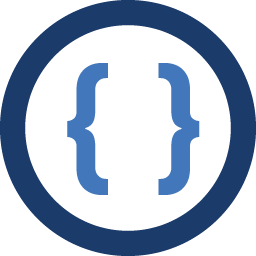
Admin
Updated on July 23, 2022Comments
-
Admin almost 2 years
so I am trying to insert the character, which i got from a string, to another string. Here I my actions: 1. I want to use simple:
someString.insert(somePosition, myChar);
2. I got an error, because insert requires(in my case) char* or string
3. I am converting char to char* via stringstream:stringstream conversion; char* myCharInsert; conversion << myChar //That is actually someAnotherString.at(someOtherPosition) if that matters; conversion >> myCharInsert; someString.insert(somePosition, myCharInsert);
4. Everything seems to be compiling successfully, but program crashes the gets to
conversion >> myCharInsert;
line.
5.I am trying to replace char* with string:
stringstream conversion; char* myCharInsert; conversion << myChar //That is actually someAnotherString.at(someOtherPosition) if that matters; conversion >> myCharInsert; someString.insert(somePosition, myCharInsert);
Everything seems to be OK, but when
someAnotherString.at(someOtherPosition)
becomes space, program crashes.So how do I correctly do this?