C Macro Token Concatenation involving a variable - is it possible?
Solution 1
I don't think what you're trying to do is possible. C macros are really preprocessor macros that are expanded before compilation. The variable port
, doesn't get set until runtime.
Solution 2
It is impossible. C preprocessors work by processing tokens, and they do not do any resolution or substitution that would require knowledge of the mechanics of the language (except basic arithmetic involving integer literals, off the top of my head). Consider, for example, the docs for GCC's preprocessor regarding tokenisation. Only a compiler will know what to do with the variable "port."
One solution is to do something like:
#define PxDIR(var, portnum) do { \
var = portnum; \
P##portnum##DIR |= blah; \
} while(0)
...and later...
int port;
PxDIR(port, 2);
I leave it to you to make this not as ugly or hacky as it is here (and more general, depending on what you need) :)
Solution 3
... or just make PORT also a macro:
#define PORT 2
#define GLUER(x,y,z) x##y##z
#define PxDIR(x) GLUER(P,x,DIR)
int main() {
PxDIR(PORT) |= 0x01;
return 0;
}
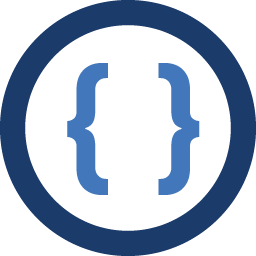
Admin
Updated on July 24, 2022Comments
-
Admin almost 2 years
I'm trying to define a macro to generate a token name, containing a variable.
Basically, what I'm trying is this:
#define GLUER(x,y,z) x##y##z #define PxDIR(x) GLUER(P,x,DIR) int main() { int port; port = 2; PxDIR(port) |= 0x01; }
I'm hoping to generate the token P2DIR in the above statement, but according to my compiler output, it's generating the token PportDIR, which is NOT what I wanted. Any help here? Or is what I'm attempting to do impossible?
-
Admin over 13 yearsThanks, that's what I was thinking. It'd been a while since I've done straight C.
-
Admin over 13 yearsThanks. I was looking into it as a way to reduce code bloat in an embedded project, but it looks as though it's impossible to use a variable to solve this problem. Ah well. Thanks again!
-
detly over 13 years@user438605 Indeed — although you may want to look at the X Macro Idiom for repeated code generation.