C++ pointer to boolean value
Solution 1
testbool_holder.state
returns true if state is not a null pointer
*(testbool_holder.state)
returns the value of the bool pointed to by state
Try this for a more C++ solution
class BoolHolder
{
public:
BoolHolder(bool* state) : state(state) {}
bool GetState() const { return *state; } // read only
bool& GetState() { return *state; } // read/write
private:
bool* state;
}
bool testbool = false;
BoolHolder testbool_holder(&testbool);
if (testbool_holder.GetState()) { .. }
Remove the second getter if you only want to allow read access (and maybe change the pointer to const bool *
) If you want both, then you need both getters. (This is because read/write can't be used to read on a const object).
Solution 2
It's right. What you have is not the value of the boolean but a pointer to the boolean. You must dereference the pointer to obtain the value of the bool itself. Since you have a pointer, it will contain an address which is an integer. Since in C and C++ all non zero integers are true, you will get true.
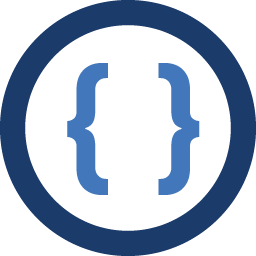
Admin
Updated on March 30, 2020Comments
-
Admin about 4 years
I'm having problems trying to implement a class which contains a pointer to a boolean...
class BoolHolder { public: BoolHolder(bool* _state); bool* state; } BoolHolder::BoolHolder(bool* _state) { state = _state; } bool testbool = false; BoolHolder testbool_holder( &testbool );
If I do this, testbool_holder.state always reports that it is true, no matter whether testbool itself is true or false
What am I doing wrong? I just want the class to be able to maintain an up to date value for testbool but I don't know how to effect this. Thanks