C++ "Variable not declared in this scope" - again
Solution 1
void setName( char* _name )
{
name = _name;
}
should be
void Animal::setName( char* _name )
{
this->name = _name;
}
You need to have Animal::
if you use the this
parameter. Without Animal::
it thinks you are just creating a new global function called setName
Solution 2
The way you have written the code setName
is a free function, not a member function. For this reason the compiler can't resolve name
.
You'll have to change setName to this:
void Animal::setName( char* _name )
{
name = _name;
}
Solution 3
The hint was "non-member function".
You need to make the function into a member function:
void Animal::setName( char* _name )
{
name = _name;
}
Solution 4
"It compiles if I comment out the setName method"
You don't have a "setName method" in your program (referring to the problematic definition). You defined a completely independent global function called setName
, which is not a "method" of anything. If you want to define a method, i.e. a member function of a class, you have to refer to it using the class_name::method_name
format. That would be Animal::setName
in your case.
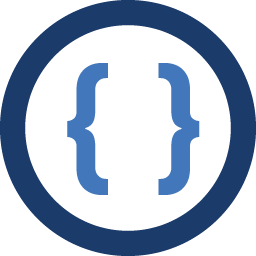
Admin
Updated on March 29, 2020Comments
-
Admin about 4 years
I guess this is a really simple question and, probably, one that has been answered several times over. However, I really do suck at C++ and have searched to no avail for a solution. I would really appreciate the help.
Basically:
#ifndef ANIMAL_H #define ANIMAL_H class Animal { public: void execute(); void setName(char*); Animal(); virtual ~Animal(); private: void eat(); virtual void sleep() = 0; protected: char* name; }; class Lion: public Animal { public: Lion(); private: virtual void sleep(); }; class Pig: public Animal { public: Pig(); private: virtual void sleep(); }; class Cow: public Animal { public: Cow(); private: virtual void sleep(); }; #endif
Is the header file, where:
#include <iostream> #include "Animal.h" using namespace std; Animal::Animal() { name = new char[20]; } Animal::~Animal() { delete [] name; } void setName( char* _name ) { name = _name; } void Animal::eat() { cout << name << ": eats food" << endl; } void Animal::execute() { eat(); sleep(); } Lion::Lion() { name = new char[20]; } void Lion::sleep() { cout << "Lion: sleeps tonight" << endl; } Pig::Pig() { name = new char[20]; } void Pig::sleep() { cout << "Pig: sleeps anytime, anywhere" << endl; } Cow::Cow() { name = new char[20]; } void Cow::sleep() { cout << "Cow: sleeps when not eating" << endl; }
is the C file. As you can see, really simple stuff, but, I get the: "error: ‘name’ was not declared in this scope" whenever I try to compile.
It compiles if I comment out the setName method. Iv tried setting 'name' to public and still get the same error. I have also tried using "this->name = _name" in setName(), which results in "invalid use of ‘this’ in non-member function".
I don't know what else to search for. Thanks in advance.