C++ Reading and Editing pixels of a bitmap image
Solution 1
But if by "bitmap" you really meant a bitmap (that is, a raw image file with nothing but uncompressed pixel data), it's rather easy:
char *filename = "filename";
FILE *input = fopen(filename, "rb+");
if (!input)
{
printf("Could not open input file '%s'\n", filename);
system("PAUSE");
return 1;
}
fseek(input, 0, SEEK_END);
size_t size = ftell(input);
rewind(input);
char *buffer = (char *) malloc(size);
if (!buffer)
{
printf("Out of memory, could not allocate %u bytes\n", size);
system("PAUSE");
return 1;
}
if (fread(buffer, 1, size, input) != size)
{
printf("Could not read from input file '%s'\n", filename);
free(buffer);
fclose(input);
system("PAUSE");
return 1;
}
fclose(input);
// we loaded the file from disk into memory now
for (size_t i = 0; (i + 3) < size; i += 4)
{
if (buffer[i + 0] == 0xFF && buffer[i + 1] == 0x00 && buffer[i + 2] == 0x00 && buffer[i + 3] == 0x00)
{
printf("Pixel %i is red\n", (i / 4));
}
}
free(buffer);
Sorry, this is C style, but you can easily change the allocation to C++ style. Personally I like the C way of reading files though. Typically you will want the if statement to check if the pixel is red to be a macro / function. This will make the if statement like "if (compare_4bytes(buffer, 0xFF000000))".
Also, this assumes the file loaded consists entirely of raw pixel data. If this is not the case, ie. you read a .bmp / .png / .jpg or something else, you must first load and then convert the file in memory to raw pixel data. This would be done at the comment saying "we loaded the file from disk into memory now".
BMP is certainly doable (it's almost raw pixel data already), but most other types need A LOT of work / research. They use often complex compression algorithms. I suggest you'd use a library for this, just for converting it to raw pixel data.
Corona is a good (small) one (http://corona.sourceforge.net/), or DevIL (http://openil.sourceforge.net/) or thousand others :)
Solution 2
If by "bitmap" you mean BMP, Wikipedia has a pretty comprehensive description of the bits and bytes
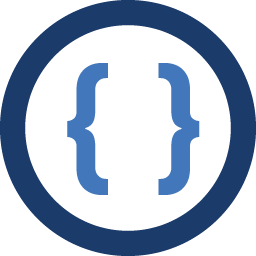
Admin
Updated on July 11, 2022Comments
-
Admin almost 2 years
I'm trying to create a program which reads an image in (starting with bitmaps because they're the easiest), searches through the image for a particular parameter (i.e. one pixel of (255, 0, 0) followed by one of (0, 0, 255)) and changes the pixels in some way (only within the program, not saving to the original file.) and then displays it.
I'm using Windows, simple GDI for preference and I want to avoid custom libraries just because I want to really understand what I'm doing for this test program.
Can anyone help? In any way? A website? Advice? Example code?
Thanks