C# Removing trailing '\0' from IP Address string
Solution 1
IPAddress.TryParse(msg.nonTargetIp.Replace("\0", String.Empty).Trim(), out ip);
Alternatively reading up on Trim() you could do the following which is probably quicker:
IPAddress.TryParse(msg.nonTargetIp.TrimEnd(new char[] { '\0' } ), out ip);
Solution 2
The other examples have shown you how to trim the string - but it would be better not to get the "bad" data to start with.
My guess is that you have code like this:
// Bad code
byte[] data = new byte[8192];
stream.Read(data, 0, data.Length);
string text = Encoding.ASCII.GetString(data);
That's ignoring the return value of Stream.Read
. Your code should be something like this instead:
// Better code
byte[] data = new byte[8192];
int bytesRead = stream.Read(data, 0, data.Length);
string text = Encoding.ASCII.GetString(data, 0, bytesRead);
Note that you should also check whether the stream has been closed, and don't assume you'll be able to read all the data in one call to Read
, or that one call to Write
at the other end corresponds to one call to Read
.
Of course it's entirely possible that this isn't the case at all - but you should really check whether the other end is trying to send these extra "null" bytes at the end of the data. It sounds unlikely to me.
Solution 3
The Trim method is more efficient in comparison to the Replace method.
msg.nonTargetIP.Trim(new [] { '\0' });
Solution 4
You could try string replacement instead of trimming:
IPAddress.TryParse(msg.nonTargetIP.Replace("\0", ""), out ip);
Solution 5
You could just use TrimEnd()
for this:
bool isIPAddress = IPAddress.TryParse(msg.nonTargetIP.nonTargetIP.TrimEnd('\0'), out ip);
Note however that the example you give contains an illegal IP address and will never successfully parse regardless (300 is > 255, each decimal number must be in the range from 2 to 255).
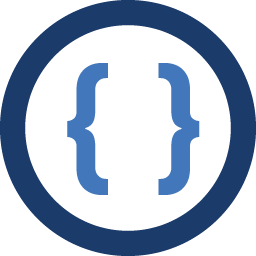
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I received a string over TCP socket. And this string looks something like this:
str = "10.100.200.200\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0";
How should I parse this into an IPAddress? If I do this,
IPAddress.TryParse(msg.nonTargetIP.Trim(), out ip);
it fails to parse.
What is simplest way to remove those trailing null's?
-
Admin almost 13 yearsI am already using the "better code" version. But issue is that I working with the byte[] data and parsing that.
-
Jon Skeet almost 13 years@ceonikka: Are you in control of the server? Is this garbage data expected?
-
Admin almost 13 yearsI am the server and clients are mostly Java/C++ implementations.
-
Jon Skeet almost 13 years@ceonikka: Okay, so what does the protocol say about what they should be sending you? How are you meant to know where the string ends?
-
Admin almost 13 yearsLegacy Protocol says null terminated strings. On side note, I ran various trim/replace solutions under debugger and I don't seems to see any change in string. Though it works fine for IPAddress!!! Elsewhere may be I should just use split() method, to grab everything before '\0'??
-
Jon Skeet almost 13 yearsTrim/Replace solutions definitely will work - but remember they won't change the existing string; they'll return a new string. I wouldn't use
Split
- I'd write one method to do this, which would useIndexOf
to find the first\0
and return everything before that.