C# replace all occurrences of a character with just a character
22,617
Solution 1
There is a solution with Regex.Replace
method:
private static string TruncatePercents(string input)
{
return Regex.Replace(input, @"%+", "%");
}
Solution 2
Use this if you want to replace one or more non-word characters with the single same non-word character.
string result = Regex.Replace(str, @"(\W)+", "$1");
Solution 3
This'll sort it:
string ProcessText(string s)
{
return Regex.Replace(s, @"%+", "%");
}
Edit Got beaten to the punch, so to make mine slightly different and to offer scope for varying delimeters:
private void button1_Click(object sender, EventArgs e)
{
label1.Text = ProcessText(textBox1.Text, "%", "#");
}
string ProcessText(string s, string delim, string replaceWith)
{
return Regex.Replace(s, @""+ delim + "+", replaceWith);
}
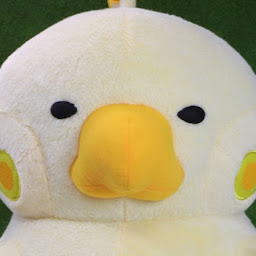
Author by
Dhinnesh Jeevan
Updated on February 17, 2020Comments
-
Dhinnesh Jeevan about 4 years
Let's say I have this string,
"%%%%%ABC"
, I would like to replace all the"%"
with just one"%"
, so it should be"%ABC"
.If its
"%%%A%%B%%C%%"
, it should be"%A%B%C%"
How should I do this?