C++ Reverse Array
37,838
Solution 1
Despite this looking quite homeworky, may I suggest:
void reverse(char word[])
{
int len=strlen(word);
char temp;
for (int i=0;i<len/2;i++)
{
temp=word[i];
word[i]=word[len-i-1];
word[len-i-1]=temp;
}
}
or, better yet, the classic XOR implementation:
void reverse(char word[])
{
int len=strlen(word);
for (int i=0;i<len/2;i++)
{
word[i]^=word[len-i-1];
word[len-i-1]^=word[i];
word[i]^=word[len-i-1];
}
}
Solution 2
Since this is homework, I'll point you toward a solution without just giving you the answer.
Your reverse
function can modify the word
that is passed in. One thing you'll need to know is how long the word is (so you'll know how many letters to reverse), you can get this from the strlen()
function. If you're not permitted to use pointers, then you can use a local int
index variable.
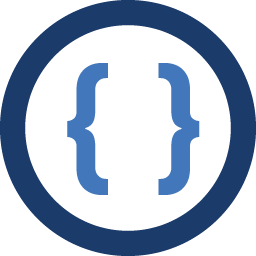
Author by
Admin
Updated on December 27, 2021Comments
-
Admin over 2 years
In C++, I need to:
- Read in a string from user input and place it into a char array [done]
- Then pass that array to a function [done]
- The function is supposed to reverse the order of characters [problem!]
- Then, back in the
main()
, it displays that original array with the newly reversed characters.
I'm having trouble creating the function that actually does the reversing because I have some restrictions:
- I cannot have any local array variables.
- No pointers either
My function is only passing in the original array ie:
void reverse(char word[])
EDIT: Here's my code base so far:
void reverse(char word[]); void main() { char word[MAX_SIZE]; cout << endl << "Enter a word : "; cin >> word; cout << "You entered the word " << word << endl; reverse(word); cout << "The word in reverse order is " << word << endl; } void reverse(char myword[]) { int i, temp; j--; for(i=0;i<(j/2);i++) { temp = myword[i]; myword[i] = myword[j]; myword[j] = temp; j--; } }
-
Greg Hewgill almost 15 yearsThis doesn't return the reversed array, as requested by the question.
-
Tom almost 15 years@Greg Where does it say that must return reversed array?
-
Greg Hewgill almost 15 years@Tom: "back in the main(), it displays that original array with the newly reversed characters"
-
Tom almost 15 years@Greg: Question states: void reverse(char word[])
-
Admin almost 15 yearsCorrect. Below is what I have so far. It doesn't actually build unless I pass an int along with the char (for j). When I do that though, it only swaps the first and last characters. void reverse(char word[]); void main() { char word[MAX_SIZE]; cout << endl << "Enter a word : "; cin >> word; cout << "You entered the word " << word << endl; reverse(word); cout << "The word in reverse order is " << word << endl; } void reverse(char myword[]) { int i,temp; j--; for(i=0;i<(j/2);i++) { temp=myword[i]; myword[i]=myword[j]; myword[j]=temp; j--; } }
-
Tom almost 15 yearsIve put this into your question. Do that in the future, so that everyone sees your advance (edit your question)
-
Admin almost 15 yearsSo, should I convert my char array into a string to use the strlen() function?
-
Admin almost 15 yearsThanks Dave, this really helps.
-
Greg Hewgill almost 15 yearsA string is exactly the same as a character array. You can call strlen(word).
-
Admin almost 15 yearsThanks Greg, I was getting thrown off by a 'warning' that it was giving me. It was building just fine.
-
Void almost 15 years@dazedandconfused: I've seen this question in C++ related job interviews. I agree with Greg. It is important you understand how his solution works. On a related note, in the real world one could simply use the standard reverse algorithm to reverse the order of characters in the array, i.e. std::reverse(word, word + strlen(word)). I wouldn't give that simple solution to your teacher, however. :)
-
Yacoby over 14 yearsUsing XOR will be far slower than swapping using a temp object.
-
Admin about 11 yearsThis isn't really what the author of this question was asking for - they wanted to reverse an array.