C++ Sorting Custom Objects in a list
17,219
Since the list contains pointers, rather than objects, you'll have to provide a custom comparator to compare the objects they point to. And since you're using a list
, you have to use its own sort
method: the generic std::sort
algorithm only works on random-access sequences.
EventList.sort([](Event * lhs, Event * rhs) {return lhs->time < rhs->time;});
or, if you're stuck in the past and can't use lambdas:
struct CompareEventTime {
bool operator()(Event * lhs, Event * rhs) {return lhs->time < rhs->time;}
};
EventList.sort(CompareEventTime());
If the list contained objects (as it probably should), then it might make sense to provide a comparison operator instead:
bool operator<(Event const & lhs, Event const & rhs) {return lhs.time < rhs.time;}
std::list<Event> EventList;
//...
EventList.sort();
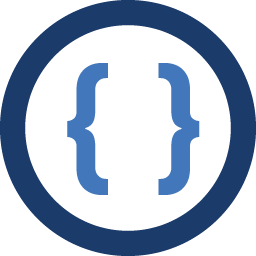
Author by
Admin
Updated on June 10, 2022Comments
-
Admin almost 2 years
I am having trouble sorting a list of custom class pointers. The class I need to sort are events. These get assigned a random time and I need to do them in the right order.
#include <list> Class Event{ public: float time; // the value which I need to sort them by int type; // to indicate which event i'm dealing with Event(float tempTime, int tempType) { time = tempTime; type = tempType; } int main(){ std::list<Event*> EventList; list<Event*>::iterator it; .........
If you could help me sort this out it would be much appreciated! I've been stuck on this for hours now.
Thanks!