C++ string in classes
Solution 1
using namespace std;
That's what's going on.
You don't have std::
prefixing the string in your class. Everything in the standard library is in the namespace std
.
It is generally regarded as bad practice to use using namespace std;
, by the way. For more information on why and what to do instead, check out this question: Using std Namespace.
Solution 2
The string class is defined in the std namespace. You should chenge the class to this:
class Language
{
public:
private:
std::string str;
};
It is also possible, but not recommended to add this to the top of the header file:
using namespace std;
Solution 3
string is in namespace std, and you need to qualify it fully inside your header file:
#include <string>
class Language
{
public:
private:
std::string str;
};
Do not use using namespace std;
or similar in header files.
Solution 4
You should refer to it as std::string;
Solution 5
It looks to me like you're missing the all-important (with a hint of sarcasm) using namespace std;
line. Either add that in before your class, or explicitely use std::string str
. I'd recommend against adding the using namespace std;
line in a header file, as it would pollute the mainspace for any file that includes it.
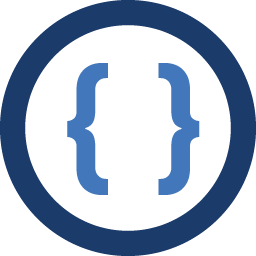
Admin
Updated on September 03, 2020Comments
-
Admin over 3 years
I know this is quite a ridiculous question but this is quite confusing and irritating, as something that should work simply is not. I'm using Code Blocks with the GCC compiler and I am trying to simply create a string variable in my class
#ifndef ALIEN_LANGUAGE #define ALIEN_LANGUAGE #include <string> class Language { public: private: string str; }; #endif
Strange enough, my compiler halts me with an error saying this:
C:\Documents and Settings\...|11|error: `string' does not name a type| ||=== Build finished: 1 errors, 0 warnings ===|
For some reason, it is unable to find the class "string" which for some reason, my main.cpp is able to detect "#include " while my language class is not able for some reason.
This is the main I wrote quickly just to see it main itself is able to see the string file:
//main.cpp #include <iostream> #include <string> #include "alien_language.h" using namespace std; int main() { string str; return 0; }
Does anyone know what's going on?
-
Admin over 14 yearsThank you, I've completely forgot that string the std:: prefixing, I'm used to not putting using namespace std; in my class files, but doing so in my main.cpp
-
sbi over 14 yearsAh, someone not recommending
using namespace std;
! +1 -
Mogi almost 4 yearswhy not to
using namespace std;
in header files? -
Mogi almost 4 yearsnevermind, find the appropriate answer after googling: cplusplus.com/forum/beginner/25538 leaving these comments for future confusions