C++: Struct Naming Standards
Solution 1
Find a style that you like and stick to it. Its as simple as that. I do it like this:
struct Foo
{
int bar;
char baz;
Foo foo;
};
Struct's and classes have their first character capitalized, variables inside don't.
But again, this is my style, find a style that suits you and stick to it. Nothing more to be said.
Solution 2
This is complete list for Naming conventions that I consider to be very complete:
- Use US-English for naming identifiers.
- Use Pascal and Camel casing for naming identifiers.
- Do not use Hungarian notation or add any other type identification to identifiers.
- Do not prefix member fields.
- Do not use casing to differentiate identifiers.
- Use abbreviations with care.
- Do not use an underscore in identifiers.
- Name an identifier according to its meaning and not its type.
- Name namespaces according to a well-defined pattern.
- Do not add a suffix to a class or struct name.
- Use a noun or a noun phrase to name a class or struct.
- Prefix interfaces with the letter I.
- Use similar names for the default implementation of an interface.
- Suffix names of attributes with Attribute.
- Do not add an Enum suffix to an enumeration type.
- Use singular names for enumeration types.
- Use a plural name for enumerations representing bitfields.
- Do not use letters that can be mistaken for digits, and vice versa.
- Add EventHandler to delegates related to events.
- Add Callback to delegates related to callback methods.
- Do not add a Callback or similar suffix to callback methods.
- Use a verb (gerund) for naming an event.
- Do not add an Event suffix (or any other type-related suffix) to the name of an event.
- Use an –ing and –ed form to express pre-events and post-events.
- Prefix an event handler with On.
- Suffix exception classes with Exception.
- Do not add code-archive related prefixes to identifiers.
- Name DLL assemblies after their containing namespace.
- Do not add MR building block prefixes to source files.
- Use Pascal casing for naming source files.
- Name the source file to the main class
- Only use the
this
. construction.
This is for C# but also apply for Java/C++. If you want to standarize your code I recommend you to see the HIGH·INTEGRITY C++ CODING STANDARD MANUAL.
For casing identifiers you should do it as follow:
- Class, Struct (Pascal, eg: AppDomain)
- Enum type (Pascal, eg: ErrorLevel)
- Enum values (Pascal, eg: FatalError)
- Event (Pascal, eg: ValueChange)
- Exception class Pascal, eg: WebException)
- Field (camel, eg: listItem)
- Const Field Pascal, eg: MaximumItems)
- Read-only Static Field Pascal, eg: RedValue)
- Interface (Pascal, eg: IDisposable)
- Method (Pascal, eg: ToString)
- Namespace (Pascal, eg: System.Drawing)
- Parameter (camel, eg: typeName)
- Property (Pascal, eg: BackColor)
Solution 3
Harmonise your style with the people around you -- within reason, it doesn't matter which naming scheme you use for structs and variables, provided everyone on your team is doing a consistent thing.
Personally, I name structs like this:
FunkyContainer
And variables like this:
ratherUsefulVariable
But you have to adapt what you do to fit the house style, or you'll ruin the consistency of your team's code.
As a side note, you don't have "structs inside objects" -- you can have nested structs, which are structs inside structs, but that's not the same thing. An object is an instance of a struct, not the struct itself. (Objects can also be instances of classes, obviously, but that's irrelevant for the purpose of making the distinction here.)
Solution 4
Any naming standard I'm familiar with are for the platform, library, etc. For example, Windows SDK has a standard, the CRT has a standard, and MFC has a standard.
I've seen no standard for C++. Perhaps you should look at what naming conventions others are using on your particular platform.
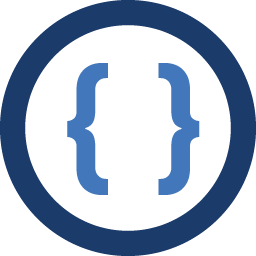
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
How should I name structs and their variables? This include structs inside objects...
Thanks.
-
Admin over 13 yearsI'm following this standard for the most part: possibility.com/Cpp/CppCodingStandard.html
-
Jonathan Wood over 13 yearsThen that's your answer. In the end, all that matters is that you and the people you work with are comfortable with the naming standards used.
-
Admin over 13 yearsSorry I wasn't clear, by "object" I meant an instance of a class.
-
Admin over 13 yearsThat standard doesn't talk about structs, so that is why I asked the question.
-
ArBR over 13 yearsYou shoul add comments about whay you're down-voting. So I can understand whats wrong with this.
-
Admin over 13 yearsc'mon, that is not what I'm looking for...
-
Stuart Golodetz over 13 years@Jay: Same comment applies there -- you don't have nested structs inside objects, you have nested structs inside classes.
-
Miles Rout over 6 yearscamel case is certainly not standardised.
-
MirkoBanchi over 5 yearsI think this is not valid C++ code.
-
Bat over 5 yearsStyling should be taken more seriously. Getting used to arbitrary styling convention is a serious mistake. It's better to follow the standard styling conventions, eg. search for Google c++ convention.