c# System.InvalidOperationException
Solution 1
The InvalidOperationException
that you receive will likely have the inner exception "The calling thread cannot access this object because a different thread owns it".
For the sake of debugging you can quickly set CheckForIllegalCrossThreadCalls
to false. This will stop the run-time from throwing you an exception, however just because you're not receiving the exceptions does not mean that they are not happening so this is a bad practice for distributed software but very handy for debugging.
In order to resolve your issue you need to invoke the code that interacts with the RichTextBox
on the UI Thread aka the thread the control was created on and the only thread that is allowed to run code that interacts with the control.
To invoke the code on the UI Thread you can use the following, succinct statement:
richTextRxMessage.BeginInvoke(new MethodInvoker(() => richTextRxMessage.Text = szData));
Very simply, all you are doing here is passing the statement you wish to invoke on the UI Thread by means of a delegate.
Solution 2
Here's a function I use in some of my stuff;
public static void InvokeEx<T>(this T @this, Action<T> action) where T : ISynchronizeInvoke
{
if (@this.InvokeRequired)
{
@this.Invoke(action, new object[] { @this });
}
else
{
action(@this);
}
}
It's pretty much a nice little method for dealing with any sort of invoke, and cuts down the lines of code when you're using it. To use it, simply use this;
Control.InvokeEx(f => control.DoStuff());
So for example, you might use;
richTextRxMessage.InvokeEx(f => richTextRxMessage.Text = szData);
Edit: As others have said, this error is more than likely due to accessing a control on a thread other than the one it was created on, which is why the invokes are required.
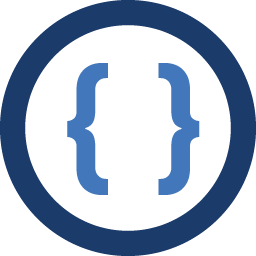
Admin
Updated on March 15, 2020Comments
-
Admin about 4 years
General
I'm trying to write a very simple TCPIP client server in C# to connect to an IP Address, with a port number, and ask quite simple line commands, and then place the replies in a Gridbox, graph or other display option.
I have looked online and found a down loadable utility that does just this, written by Jayan Nair, and this appears to send the message correctly, and receive the reply ok.
The problem comes when I try and load the reply data into a richtext or GridView.
The error message I'm getting is :- System.InvalidOperationException
I have asked Microsoft Forums, and they have given me a very complicated, ambiguous and overly involved indication as to what I should do, and this involves something called INVOKE and BeginInvoke, and they whole things seems to be a project in it;s own right.
What I'm after is just an example that works, without being too complicated.
Here's the code :-
try { SocketPacket theSockId = (SocketPacket)asyn.AsyncState; int iRx = theSockId.thisSocket.EndReceive(asyn); char[] chars = new char[iRx + 1]; System.Text.Decoder d = System.Text.Encoding.UTF8.GetDecoder(); int charLen = d.GetChars(theSockId.dataBuffer, 0, iRx, chars, 0); // System.String szData = new System.String(chars); richTextRxMessage.Text = szData; // fails //textBox1.Text = szData; // also fails WaitForData(); }
and here's the error message :-
base {System.Windows.Forms.TextBoxBase} = {Text = '((System.Windows.Forms.RichTextBox) (((System.Windows.Forms.RichTextBox)(richTextRxMessage)))).Text' threw an exception of type 'System.InvalidOperationException'}
Additional information is :- szData contains about 6300 characters, including tabs (9) and returns (13), and is consistant with the message sent from the server I've also tried it with using a textbox instead of richtext, same result
For those interested
Here's the Microsoft link
- Here is just 2 of the code amendments that I've tried, both fail on the same error condition
- I think what I need to do is start C# at a much lower level and not just jump in and hope for the best
public void OnDataReceived(IAsyncResult asyn) { int InputRx; int charLen; char[] Inputchars; System.Text.Decoder InputDecode; System.String szData; bool IfInvokeRequired; try { SocketPacket theSockId = (SocketPacket)asyn.AsyncState; InputRx = theSockId.thisSocket.EndReceive(asyn); // get size of input array Inputchars = new char[InputRx + 1]; // put i char array InputDecode = System.Text.Encoding.UTF8.GetDecoder(); charLen = InputDecode.GetChars(theSockId.dataBuffer, 0, InputRx, Inputchars, 0); szData = new System.String(Inputchars); IfInvokeRequired = richTextRxMessage.InvokeRequired; if (IfInvokeRequired == true) { richTextRxMessage.Invoke((MethodInvoker)delegate { this.Text = szData; });// fails richTextRxMessage.BeginInvoke(new MethodInvoker(() => richTextRxMessage.Text = szData));//fails as well }
-
Sinatr about 11 yearsYou can do
dirtyImage.Invoke((MethodInvoker)delegate {Visible = RigX.IsDirty; Refresh();});
. Also I personally would create a...Invoked
method for anything what is more than 1 command because you will have just 1 peace of code to check/change. -
Nevyn about 11 yearsolder code, I know that now...but never have had permission to go back in and refactor it. Damn business rules and permission required production environments :)