C# - The ObjectContext instance has been disposed and can no longer be used for operations that require a connection
Solution 1
The problem is solved. I get help from my friend. Its about unclosed connection. Its my fault. I didn't mention it.
In PersonIndexVM()
, I created People = new List<Person>();
Person class is created by entity framework. It is related with database. When I create a model
that is an object of PersonIndexVM()
in GetPeople()
method and return this model
object as a json, model object try to reach User class informations after the connection closed. And I'm getting this error. To solve this problem:
Closing the lazy loading to prevent reaching User information.
dc.Configuration.LazyLoadingEnabled = false;
Creating another class not related with database and return its object as Json.
Solution 2
You can strongly type your inclusion, which will give you hints as to whether or not your object structure is correctly related. This solves any "magic strings" issues, such as your table being named Users instead of User inside of your EF context, after including DbExtension
.
using System.Data.Entity.DbExtension;
model.People = dc.People.Include(c => c.User).ToList();
However, if you are using ObjectContext
instead of DbContext, you may be stuck with magic strings. That said, EF will "pluralize" most names, so your table is most likely named "Users". Check to see if the table exists as dc.Users
and, if it does, change your magic string to match.
model.People = dc.People.Include("Users").ToList();
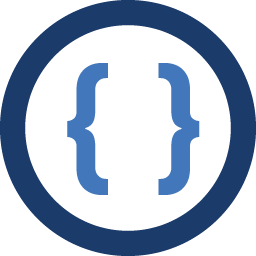
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I'm sorry for the repetition. I'm heading with a same problem but still could not handle it. I'm using Angularjs framework and Asp.net mvc.
My person class:
public partial class Person { public int PersonID { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public string Telephone { get; set; } public string Adress { get; set; } public int UserID { get; set; } public virtual User User { get; set; } }
My User class:
public partial class User { public User() { this.People = new HashSet<Person>(); } public int UserID { get; set; } public string Username { get; set; } public string Password { get; set; } public string FullName { get; set; } public string Email { get; set; } public string Gender { get; set; } public virtual ICollection<Person> People { get; set; } }
My js code:
$http.get('/Data/GetPeople', { params: { UserID: "1" } }).success(function (data) { $scope.model = data; });
I'm trying to get records from my database:
public ActionResult GetPeople(int UserID) { using (PersonEntities dc = new PersonEntities()) { var model = new PersonIndexVM(); model.People = dc.People.Where(b => b.UserID == UserID).ToList(); //model.People = dc.People.Include("User").ToList(); return Json(model, JsonRequestBehavior.AllowGet); } }
As I see with debugging, I'm getting the right objects from database in GetPeople method. But after that I'm getting this error:
'The ObjectContext instance has been disposed and can no longer be used for operations that require a connection.'
I tried to Eagerly load:
model.People = dc.People.Include("User").Where(b => b.UserID == UserID).ToList();
Still getting the same error.It would be a pleasure if you help me.