C# Using Assembly to call a method within a DLL
28,019
//Assembly1.dll
using System;
using System.Reflection;
namespace TestAssembly
{
public class Main
{
public void Run(string parameters)
{
// Do something...
}
public void TestNoParameters()
{
// Do something...
}
}
}
//Executing Assembly.exe
public class TestReflection
{
public void Test(string methodName)
{
Assembly assembly = Assembly.LoadFile("...Assembly1.dll");
Type type = assembly.GetType("TestAssembly.Main");
if (type != null)
{
MethodInfo methodInfo = type.GetMethod(methodName);
if (methodInfo != null)
{
object result = null;
ParameterInfo[] parameters = methodInfo.GetParameters();
object classInstance = Activator.CreateInstance(type, null);
if (parameters.Length == 0)
{
result = methodInfo.Invoke(classInstance, null);
}
else
{
object[] parametersArray = new object[] { "Hello" };
result = methodInfo.Invoke(classInstance, parametersArray);
}
}
}
}
}
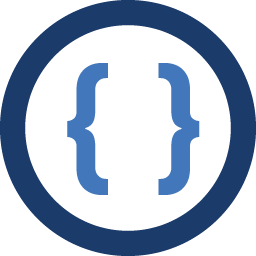
Author by
Admin
Updated on August 01, 2020Comments
-
Admin almost 4 years
I have been reading a lot about this - I feel like I'm very close to the answer. I am simply looking to call a method from within a dll file that I have created.
For example purposes:
My DLL File:
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ExampleDLL { class Program { static void Main(string[] args) { System.Windows.Forms.MessageBox.Show(args[0]); } public void myVoid(string foo) { System.Windows.Forms.MessageBox.Show(foo); } } }
My Application:
string filename = @"C:\Test.dll"; Assembly SampleAssembly; SampleAssembly = Assembly.LoadFrom(filename); // Obtain a reference to a method known to exist in assembly. MethodInfo Method = SampleAssembly.GetTypes()[0].GetMethod("myVoid"); // Obtain a reference to the parameters collection of the MethodInfo instance.
All credits go to SO user 'woohoo' for the above snippet How to call a Managed DLL File in C#?
Now, though, I would like to be able to not only reference my Dll (and the methods inside it) but properly call the methods inside it (in this case I would like to call method 'myVoid').
Might anyone have any suggestions for me?
Thank you,
Evan