C++: Where to initialize variables in constructor
Solution 1
In short, always prefer initialization lists when possible. 2 reasons:
If you do not mention a variable in a class's initialization list, the constructor will default initialize it before entering the body of the constructor you've written. This means that option 2 will lead to each variable being written to twice, once for the default initialization and once for the assignment in the constructor body.
Also, as mentioned by mwigdahl and avada in other answers, const members and reference members can only be initialized in an initialization list.
Also note that variables are always initialized on the order they are declared in the class declaration, not in the order they are listed in an initialization list (with proper warnings enabled a compiler will warn you if a list is written out of order). Similarly, destructors will call member destructors in the opposite order, last to first in the class declaration, after the code in your class's destructor has executed.
Solution 2
Although it doesn't apply to this specific example, Option 1 allows you to initialize member variables of reference type (or const
type, as pointed out below). Option 2 doesn't. In general, Option 1 is the more powerful approach.
Solution 3
See Should my constructors use "initialization lists" or "assignment"?
Briefly: in your specific case, it does not change anything. But:
- for class/struct members with constructors, it may be more efficient to use option 1.
- only option 1 allows you to initialize reference members.
- only option 1 allows you to initialize const members
- only option 1 allows you to initialize base classes using their constructor
- only option 2 allows you to initialize array or structs that do not have a constructor.
My guess for why option 2 is more common is that option 1 is not well-known, neither are its advantages. Option 2's syntax feels more natural to the new C++ programmer.
Solution 4
Option 1 allows you to use a place specified exactly for explicitly initializing member variables.
Solution 5
Option 1 allows you to initialize const
members. This cannot be done with option 2 (as they are assigned to, not initialized).
Why must const members be intialized in the constructor initializer rather than in its body?
Related videos on Youtube
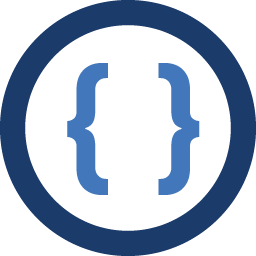
Admin
Updated on May 22, 2020Comments
-
Admin almost 4 years
Possible Duplicate:
C++ initialization listsWhat are the pros/cons of initializing variables at option 1 vs option 2?
class MyClass { public: MyClass( float f, char a ); private: float mFloat; char mCharacter; bool mBoolean; int mInteger; }; MyClass::MyClass( float f, char a ) : mFloat( f ), mBoolean( true ) // option 1. { // option 2 mCharacter = a; mInteger = 0; }
Edit: Why is option 2 so common?
-
Admin almost 13 yearsno longer a duplicate with the added edit. since option 1 is apparently better, I am curious why option 2 is so common.
-
Manu Vats almost 13 yearsIt may be a duplicate, but it seems no answer on the other question is exhaustive.
-
-
0fnt over 11 yearsThanks for the informative answer. Re your point about option 2, is that the standard or is that some specific compiler? I don't suppose it would be too hard for a compiler to optimize the initial rights away. I prefer option 2 because its cleaner.
-
Rich about 11 yearsShouldn't a compiler be able to optimise out the unnecessary automatic initialisation? I mean, C# and Java can do it, so should C++, right?
-
human.js over 9 yearsthanks for the link!
-
duleshi over 8 yearsThis is called "initialization list", not "initializer list".
-
Erik Aronesty almost 8 years@0fnt,Yes, C++ optimizes out the extra initialization, this is a non-issue. and No, just because a few members must be done that way, doesn't mean all should be. Initialization lists are, IMO, ugly and hard to read.
-
Erik Aronesty almost 8 yearsRight, but he has no reference types. So Option 2 is fine.
-
mwigdahl almost 8 yearsIn his specific example (which seems contrived rather than taken from real-world code), yep! In the more general case, Option 1 is very often superior, which is what I was getting at. I'll edit to make it more clear.
-
Ruslan over 7 years@Joey even though for primitive types it's true, this cannot be true for user types for which the compiler doesn't know what the default constructor does (e.g. defined in another compilation unit — maybe even shared library).