Calculate the start and end date of a week given the week number and year in C# (based on the ISO specification)
Solution 1
You can use the Calendar.GetWeekOfYear
method to get the week number of a date, with the CalendarWeekRule.FirstFourDayWeek
value to specify how the weeks are determined, and DayOfWeek.Monday
to specify the first weekday. That follows the ISO specification.
Example:
int week = Calendar.GetWeekOfYear(DateTime.Today, CalendarWeekRule.FirstFourDayWeek, DayOfWeek.Monday);
To get the first date of the first week of the year, you can start from the 4th of january and go back until you find the monday:
DateTime t = new DateTime(DateTime.Today,Year, 1, 4);
while (t.DayOfWeek != DayOfWeek.Monday) t = t.AddDays(-1);
Solution 2
If you want to do it manually, have a look at this post.
Solution 3
This should work. I've used it on reporting in the past. I agree that it's not very pretty though:
DateTime GetWeekStartDate(int year, int week)
{
DateTime jan1 = new DateTime(year, 1, 1);
int day = (int)jan1.DayOfWeek - 1;
int delta = (day < 4 ? -day : 7 - day) + 7 * (week - 1);
return jan1.AddDays(delta);
}
That calculates the start date for a certain week. The end DateTime is obviously 7 days later (exclusive).
You may find this code of mine useful. It's barely documented, but it implements a few other operations on the WeekAndYear struct it defines. Plenty of room for improvement. Most notably, it defines <
and >
operators, but no others, which is pretty bad... but it should get you started.
Porting to VB6 though... Hmm, maybe not :P
Solution 4
use the Calendar.GetWeekOfYear method to get the week of the current datetime, the rest should be trivial.
For vb6 it's less trivial, you're best bet is to find a good library which does the hard work for you.
Solution 5
This will give you the start of the current week
dateAdd(DateAdd(DateInterval.Day, (Now.Day * -1), Now)
To get the end of the week add 7 days to the start of the week
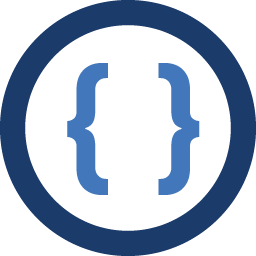
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I need to generate a report that shows the 52 weeks of a year (or 53 weeks as some years have) and their start and end dates. There is an ISO spec to do this but seems awfully complicated! Im hoping someone knows of a way to do it in C# or Visual Basic (its actually for Visual Basic 6 but I will try port it across)