Calculating the angle between two lines without having to calculate the slope? (Java)
Solution 1
The atan2
function eases the pain of dealing with atan
.
It is declared as double atan2(double y, double x)
and converts rectangular coordinates (x,y)
to the angle theta
from the polar coordinates (r,theta)
So I'd rewrite your code as
public static double angleBetween2Lines(Line2D line1, Line2D line2)
{
double angle1 = Math.atan2(line1.getY1() - line1.getY2(),
line1.getX1() - line1.getX2());
double angle2 = Math.atan2(line2.getY1() - line2.getY2(),
line2.getX1() - line2.getX2());
return angle1-angle2;
}
Solution 2
Dot product is probably more useful in this case. Here you can find a geometry package for Java which provides some useful helpers. Below is their calculation for determining the angle between two 3-d points. Hopefully it will get you started:
public static double computeAngle (double[] p0, double[] p1, double[] p2)
{
double[] v0 = Geometry.createVector (p0, p1);
double[] v1 = Geometry.createVector (p0, p2);
double dotProduct = Geometry.computeDotProduct (v0, v1);
double length1 = Geometry.length (v0);
double length2 = Geometry.length (v1);
double denominator = length1 * length2;
double product = denominator != 0.0 ? dotProduct / denominator : 0.0;
double angle = Math.acos (product);
return angle;
}
Good luck!
Solution 3
dx1 = x2-x1; dy1 = y2-y1; dx2 = x4-x3; dy2 = y4-y3; d = dx1*dx2 + dy1*dy2; // dot product of the 2 vectors l2 = (dx1*dx1+dy1*dy1)*(dx2*dx2+dy2*dy2) // product of the squared lengths angle = acos(d/sqrt(l2));
The dot product of 2 vectors is equal to the cosine of the angle time the length of both vectors. This computes the dot product, divides by the length of the vectors and uses the inverse cosine function to recover the angle.
Solution 4
Maybe my approach for Android coordinates system will be useful for someone (used Android PointF class to store points)
/**
* Calculate angle between two lines with two given points
*
* @param A1 First point first line
* @param A2 Second point first line
* @param B1 First point second line
* @param B2 Second point second line
* @return Angle between two lines in degrees
*/
public static float angleBetween2Lines(PointF A1, PointF A2, PointF B1, PointF B2) {
float angle1 = (float) Math.atan2(A2.y - A1.y, A1.x - A2.x);
float angle2 = (float) Math.atan2(B2.y - B1.y, B1.x - B2.x);
float calculatedAngle = (float) Math.toDegrees(angle1 - angle2);
if (calculatedAngle < 0) calculatedAngle += 360;
return calculatedAngle;
}
It return positive value in degrees for any quadrant: 0 <= x < 360
You can checkout my utility class here
Solution 5
The formula for getting the angle is tan a = (slope1-slope2)/(1+slope1*slope2)
You are using:
tan a = (slope1 - slope2) / (1 - slope1 * slope2)
So it should be:
double angle = Math.atan((slope1 - slope2) / (1 + slope1 * slope2));
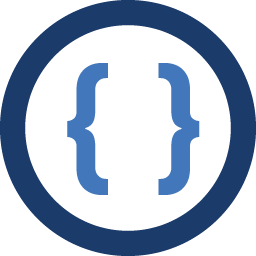
Admin
Updated on January 14, 2020Comments
-
Admin over 4 years
I have two Lines: L1 and L2. I want to calculate the angle between the two lines. L1 has points:
{(x1, y1), (x2, y2)}
and L2 has points:{(x3, y3), (x4, y4)}
.How can I calculate the angle formed between these two lines, without having to calculate the slopes? The problem I am currently having is that sometimes I have horizontal lines (lines along the x-axis) and the following formula fails (divide by zero exception):
arctan((m1 - m2) / (1 - (m1 * m2)))
where
m1
andm2
are the slopes of line 1 and line 2 respectively. Is there a formula/algorithm that can calculate the angles between the two lines without ever getting divide-by-zero exceptions? Any help would be highly appreciated.This is my code snippet:
// Calculates the angle formed between two lines public static double angleBetween2Lines(Line2D line1, Line2D line2) { double slope1 = line1.getY1() - line1.getY2() / line1.getX1() - line1.getX2(); double slope2 = line2.getY1() - line2.getY2() / line2.getX1() - line2.getX2(); double angle = Math.atan((slope1 - slope2) / (1 - (slope1 * slope2))); return angle; }
Thanks.