Call javascript function on submit form
Solution 1
This is probably due to a syntax error in your script. When you see errors like that, look into the JavaScript console of your browser.
In this case, con-password
is not a valid variable name. What JavaScript sees is:
var con - password ...
i.e. the code says "substract password
from con
". Try an underscore instead:
var con_password ...
Solution 2
Do not need to do anything extra for password matching, just add equalTo: "#Password"
to it as shown in the below example:
$(document).ready(function () {
$("#register").validate({
rules: {
"Username": {
required: true,
},
"Password": {
required: true,
minlength: 5
},
"Confirm-Password": {
required: true,
equalTo: "#Password"
},
"email": {
required: true,
}
},
messages: {
Password: {
required: "Please provide a password",
minlength: "Your password must be at least 5 characters long"
},
Confirm-Password: {
required: "Please provide a confirm password",
equalTo: "Please enter the same password as above"
}
},
submitHandler: function(form) {
// Your function call
return false; // return true will submit form
}
});
});
Working example:
<form id="register" name="register" action="" method="post">
<label for="Username"> Username </label><br>
<input type="text" class="register-control" id="Username" name="Username" placeholder="Enter Username"> <br><br>
<label for="Password"> Password </label><br>
<input type="password" class="register-control" id="Password" name="Password" placeholder="Enter Password"><br><br>
<label for="Confirm-Password"> Confirm Password </label><br>
<input type="password" class="register-control" id="Confirm_Password" name="Confirm_Password" placeholder="Confirm Password" ><br><br>
<label for="email"> Email </label><br>
<input type="email" class="register-control" id="email" name="email" placeholder="Enter Valid Email"><br><br>
<button type="submit">Submit</button>
</form>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.5.2/jquery.min.js"></script>
<script type="text/javascript" src="http://ajax.aspnetcdn.com/ajax/jquery.validate/1.8/jquery.validate.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#register").validate({
rules: {
"Username": {
required: true,
},
"Password": {
required: true,
minlength: 5
},
"Confirm_Password": {
required: true,
equalTo: "#Password"
},
"email": {
required: true,
}
},
messages: {
Password: {
required: "Please provide a password",
minlength: "Your password must be at least 5 characters long"
},
Confirm_Password: {
required: "Please provide a confirm password",
equalTo: "Please enter the same password as above"
}
},
submitHandler: function(form) {
// Your function call
return false; // return true will submit form
}
});
});
</script>
Solution 3
Maybe instead of checking if passwords matches you can add new rule in validation? something like:
... "Password": {
required: true,
minlength: 5
},
"Confirm-Password": {
required: true,
equalTo: "#Password"} ....
and for messages add:
... messages: {
"Password": "Your message",
}...
and all in all something like this: `
$(document).ready(function () {
$("Your form name").validate({
rules: {
"Username": {
required: true,
},
"Password": {
required: true,
minlength: 5
},
"Confirm-Password": {
required: true,
equalTo: "#Password"
},
"email": {
required: true,
email: true
}
}
messages: {
"Password": "Your message",
"email": "Your Message",
},
submitHandler: function (form) {
form.submit();
}
});
});`
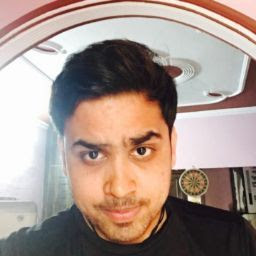
chandan111
Updated on January 22, 2020Comments
-
chandan111 over 4 years
I am trying to call JavaScript function while submitting the form.
Here is code but while submitting function not called, please suggest something and I want to show error messages using javascript method as well , how can I show error messages in validation using JavaScript.
<form id="register" name="register" onsubmit="validateForm()"> <label for="Username"> Username </label><br> <input type="text" class="register-control" id="Username" name="Username" placeholder="Enter Username"> <br><br> <label for="Password"> Password </label><br> <input type="password" class="register-control" id="Password" name="Password" placeholder="Enter Password"><br><br> <label for="Confirm-Password"> Confirm Password </label><br> <input type="password" class="register-control" id="Confirm-Password" name="Confirm-Password" placeholder="Confirm Password" ><br><br> <label for="email"> Email </label><br> <input type="email" class="register-control" id="email" name="email" placeholder="Enter Valid Email"><br><br> <button type="submit">Submit</button> </form> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.5.2/jquery.min.js"></script> <script type="text/javascript" src="http://ajax.aspnetcdn.com/ajax/jquery.validate/1.8/jquery.validate.min.js"></script> <script type="text/javascript"> $(document).ready(function () { $("#register").validate({ rules: { "Username": { required: true, }, "Password": { required: true, minlength: 5 }, "Confirm-Password": { required: true, }, "email": { required: true, } } }); }); </script>
and here is JavaScript code
function validateForm() { var password = document.forms["register"]["Password"].value; var con-password = document.forms["register"]["Confirm-Password"].value; if(password != con-password) { document.getElementById('password-error').style.visibility='visible'; alert("not matched"); } alert("matched"); }
-
chandan111 over 10 yearsthanks learan a new thing ,added but still fuction didn't work.
-
chandan111 over 10 yearsevent returnValue is deprecated. Please use the standard event.preventDefault() instead.
-
chandan111 over 10 yearsthanks it works for me, but i want to use javascript as well .
-
szpic over 10 yearsas far as I know jQuery is JS ;)
-
chandan111 over 10 yearsthanks can you please write messages part for Password and Email just for confirming the syntax.
-
Anubhav over 10 yearsYou can use submitHandler: function(form) { /*your function call*/ return false; }
-
szpic over 10 yearsadded some. But could there my some spelling mistakes. Don't have possibility at this moment to check this.
-
chandan111 over 10 years@Anubhav above trick work for me but it showing error messages in both condition when passwords are different and same .
-
Anubhav over 10 yearsNot possible, you are doing something wrong. see the documentation jqueryvalidation.org/validate. Note change hyphen(-) to underscore(_) in the names of input field!
-
Sparky over 10 years-1 for not also showing the OP that his
validateForm()
function is completely superfluous, and that the jQuery Validate plugin has asubmitHandler
built into it. -
Sparky over 10 years+1 for posting the correct answer and I agree, the OP's code could be so much better if he'd simply leverage the features of the plugin. However, it is possible to show messages upon success with the jQuery Validate plugin... one only needs to read the documentation and look at the code on Stack Overflow.