Calling a Variable from another Class
226,747
Solution 1
That would just be:
Console.WriteLine(Variables.name);
and it needs to be public also:
public class Variables
{
public static string name = "";
}
Solution 2
I would suggest to use a variable instead of a public field:
public class Variables
{
private static string name = "";
public static string Name
{
get { return name; }
set { name = value; }
}
}
From another class, you call your variable like this:
public class Main
{
public void DoSomething()
{
string var = Variables.Name;
}
}
Solution 3
You need to specify an access modifier for your variable. In this case you want it public.
public class Variables
{
public static string name = "";
}
After this you can use the variable like this.
Variables.name
Solution 4
class Program
{
Variable va = new Variable();
static void Main(string[] args)
{
va.name = "Stackoverflow";
}
}
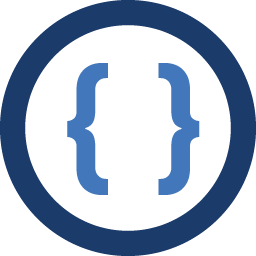
Author by
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
How can I access a variable in one public class from another public class in C#?
I have:
public class Variables { static string name = ""; }
I need to call it from:
public class Main { }
Thanks in advance for the help.
I am working in a Console App.