Calling Web API using PostAsJsonAsync
16,006
I'm assuming your issue is resolved by now, but it was probably because you were omitting the [FromBody]
attribute in your post method. You could have tried:
public Quadrilateral Post([FromBody] Quadrilateral q)
{
return new Rectangle(q);
}
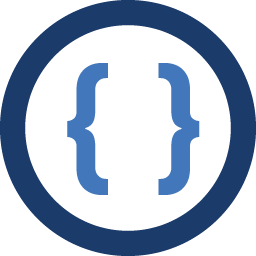
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
i am a newbie trying to call a web api method.
i have a Quadrilateral object:
public class Quadrilateral { public double SideA { get; private set; } public double SideB { get; private set; } public double SideC { get; private set; } public double SideD { get; private set; } public double AngleAB { get; private set; } public double AngleBC { get; private set; } public double AngleCD { get; private set; } public double AngleDA { get; private set; } public virtual string Name { get { return "Generic Quadrilateral"; } } public virtual string Description { get { return "A quadrilateral that has no specific name for its shape"; } } public Quadrilateral(double sideA, double sideB, double sideC, double sideD, double angleAB, double angleBC, double angleCD, double angleDA) { SideA = sideA; SideB = sideB; SideC = sideC; SideD = sideD; AngleAB = angleAB; AngleBC = angleBC; AngleCD = angleCD; AngleDA = angleDA; } }
my web api post method does something very simple. it accepts a Quadrilateral object, and returns a subtype of Quadrilateral, a rectangle:
public class Rectangle : Quadrilateral { public override string Name { get { return "Rectangle"; } } public override string Description { get { return "A quadrilateral with equal opposite sides and 4 right angles"; } } public Rectangle(Quadrilateral q) : base(q.SideA, q.SideB, q.SideC, q.SideD, q.AngleAB, q.AngleBC, q.AngleCD, q.AngleDA) { } }
the web api post method looks like this:
public Quadrilateral Post(Quadrilateral q) { return new Rectangle(q); }
i create a Quadrilateral object, assigning the members values. then i try to invoke my web api using the following code:
readonly string baseUri = "http://localhost:43798/"; readonly string controller = "api/quadrilateral"; using (HttpClient client = new HttpClient()) { client.BaseAddress = new Uri(baseUri); client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json")); HttpResponseMessage response = await client.PostAsJsonAsync(controller, quadrilateral); if (response.IsSuccessStatusCode) { return await response.Content.ReadAsAsync<Quadrilateral>(); } else { return null; } }
debugging shows that the web api post method is hit, but the Quadrilateral object now contains only 0.0 for all its double members. why is the post method not getting the object i am trying to send it?