Can't show current user location in Flutter using 'google_maps_flutter' plugin
Solution 1
Please use to plugin geolocator Plugin along with your Google_maps_flutter plugin
Dont forget to add uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" in your androidmanifest.xml
Solution 2
About the issue : The issue was with the android emulator. After updating google play service app in the emulator and then restarting emulator again fixed my issue.
For the simplified implementation, I think geolocator plugin would be great that Vasanth Vadivel already recommended here.
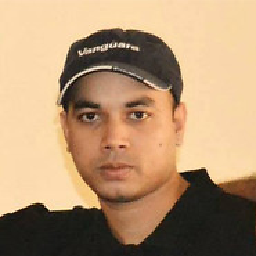
Nuibb
Updated on December 22, 2022Comments
-
Nuibb 32 minutes
I'm trying to show current user location in the map view using flutter. In iOS, it is very simple, just to add
self.mapView.showsUserLocation = YES;
and that will show the current user location in map view. But in flutter, it seems very complex and need to write lot of code as well as plugins.By the way, Is there any simple solution like native iOS for this feature in Flutter?
I was also trying to implement it in flutter way, using 'geolocator' and 'google_maps_flutter' plugin for this purpose but getting an exception in the log while running.
Installing build\app\outputs\apk\app.apk... Debug service listening on ws://127.0.0.1:51924/x_FSkxlnOcY=/ws Syncing files to device sdk gphone x86... W/one.cliffjumpe(14833): Accessing hidden method Landroid/content/Context;->getFeatureId()Ljava/lang/String; (greylist, reflection, allowed) E/flutter (14833): [ERROR:flutter/lib/ui/ui_dart_state.cc(157)] Unhandled Exception: PlatformException(ERROR_GEOCODING_COORDINATES, grpc failed, null) E/flutter (14833): #0 StandardMethodCodec.decodeEnvelope (package:flutter/src/services/message_codecs.dart:569:7) E/flutter (14833): #1 MethodChannel._invokeMethod (package:flutter/src/services/platform_channel.dart:156:18)
Here is my code snippet -
import 'package:flutter/cupertino.dart'; import 'dart:async'; import 'package:flutter/material.dart'; import 'package:geolocator/geolocator.dart'; import 'package:google_maps_flutter/google_maps_flutter.dart'; class MapScreen extends StatefulWidget { @override _MapState createState() => _MapState(); } class _MapState extends State<MapScreen> { Completer<GoogleMapController> controller1; static LatLng _initialPosition; final Set<Marker> _markers = {}; static LatLng _lastMapPosition = _initialPosition; @override void initState() { super.initState(); _getUserLocation(); } void _getUserLocation() async { Position position = await Geolocator().getCurrentPosition(desiredAccuracy: LocationAccuracy.high); List<Placemark> placemark = await Geolocator().placemarkFromCoordinates(position.latitude, position.longitude); setState(() { _initialPosition = LatLng(position.latitude, position.longitude); print('placemark : ${placemark[0].name}'); }); } _onMapCreated(GoogleMapController controller) { setState(() { controller1.complete(controller); }); } MapType _currentMapType = MapType.normal; void _onMapTypeButtonPressed() { setState(() { _currentMapType = _currentMapType == MapType.normal ? MapType.satellite : MapType.normal; }); } _onCameraMove(CameraPosition position) { _lastMapPosition = position.target; } _onAddMarkerButtonPressed() { setState(() { _markers.add( Marker( markerId: MarkerId(_lastMapPosition.toString()), position: _lastMapPosition, infoWindow: InfoWindow( title: "Pizza Parlour", snippet: "This is a snippet", onTap: (){ } ), onTap: (){ }, icon: BitmapDescriptor.defaultMarker)); }); } Widget mapButton(Function function, Icon icon, Color color) { return RawMaterialButton( onPressed: function, child: icon, shape: new CircleBorder(), elevation: 2.0, fillColor: color, padding: const EdgeInsets.all(7.0), ); } @override Widget build(BuildContext context) { return Scaffold( body: _initialPosition == null ? Container(child: Center(child:Text('loading map..', style: TextStyle(fontFamily: 'Avenir-Medium', color: Colors.grey[400]),),),) : Container( child: Stack(children: <Widget>[ GoogleMap( markers: _markers, mapType: _currentMapType, initialCameraPosition: CameraPosition( target: _initialPosition, zoom: 14.4746, ), onMapCreated: _onMapCreated, zoomGesturesEnabled: true, onCameraMove: _onCameraMove, myLocationEnabled: true, compassEnabled: true, myLocationButtonEnabled: false, ), Align( alignment: Alignment.topRight, child: Container( margin: EdgeInsets.fromLTRB(0.0, 50.0, 0.0, 0.0), child: Column( children: <Widget>[ mapButton(_onAddMarkerButtonPressed, Icon( Icons.add_location ), Colors.blue), mapButton( _onMapTypeButtonPressed, Icon( IconData(0xf473, fontFamily: CupertinoIcons.iconFont, fontPackage: CupertinoIcons.iconFontPackage), ), Colors.green), ], )), ) ]), ), ); } }