Can't Understand mHandler.obtainMessage() in Android Bluetooth sample
The main goal of Handler
is to provide interface between producer and consumer thread, here, between UI thread and worker thread. Implementation of Handler
goes into consumer thread.
In your case, you want to communicate MESSAGE_READ
between threads.
Without handler you can do nothing out of your main Activity Thread.
Therefore, look for mHandler
initiation into main Activity.
The default handler init should be like:
Handler mHandler = new Handler(){
@Override
public void handleMessage(Message msg) {
/**/
}
};
If you use Eclipse, click on your Project -> Ctrl+H -> File Search -> "Handler".
Or in Notepad++ -> Serch -> Find in files ....
[EDIT]
final int MESSAGE_READ = 9999; // its only identifier to tell to handler what to do with data you passed through.
// Handler in DataTransferActivity
public Handler mHandler = new Handler() {
public void handleMessage(Message msg) {
switch (msg.what) {
case SOCKET_CONNECTED: {
mBluetoothConnection = (ConnectionThread) msg.obj;
if (!mServerMode)
mBluetoothConnection.write("this is a message".getBytes());
break;
}
case DATA_RECEIVED: {
data = (String) msg.obj;
tv.setText(data);
if (mServerMode)
mBluetoothConnection.write(data.getBytes());
}
case MESSAGE_READ:
// your code goes here
I'm sure you must implement something like:
new ConnectionThread(mBluetoothSocket, mHandler);
sources i found here
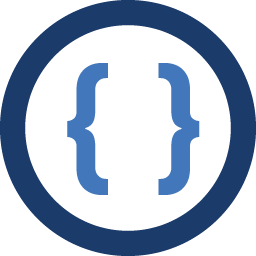
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm Working on Bluetooth rfcomm connection. There's a line in Android Sample that I can't understand and unfortunately I couldn't find a good answer in other questions and resources.
Here is the whole code:
public void run() { byte[] buffer = new byte[1024]; // buffer store for the stream int bytes; // bytes returned from read() // Keep listening to the InputStream until an exception occurs while (true) { try { // Read from the InputStream bytes = mmInStream.read(buffer); // Send the obtained bytes to the UI activity mHandler.obtainMessage(MESSAGE_READ, bytes, -1, buffer) .sendToTarget(); } catch (IOException e) { break; } } } /* Call this from the main activity to send data to the remote device */ public void write(byte[] bytes) { try { mmOutStream.write(bytes); } catch (IOException e) { } }
I can't Understand this line:
// Read from the InputStream bytes = mmInStream.read(buffer); // Send the obtained bytes to the UI activity mHandler.obtainMessage(MESSAGE_READ, bytes, -1, buffer) .sendToTarget();
mHandler
is not defined in this code and alsoMESSAGE_READ
I can't understand what does
bytes
do?I think and as mentioned in comment it sends the received Bytes to the Activity which I set as my Main Activity. Can I make a
Static TextView
in my main Activity instead of sendToTarget() to show the received message?