Can't use chrome driver for Selenium
Solution 1
You should specify the executable file path, not the directory path that contains the executable.
driver = webdriver.Chrome(executable_path=r"C:\Chrome\chromedriver.exe")
Solution 2
For Linux
1. Check you have installed latest version of chrome browser-> "chromium-browser -version"
2. If not, install latest version of chrome "sudo apt-get install chromium-browser"
3. Get the appropriate version of chrome driver from http://chromedriver.storage.googleapis.com/index.html
4. Unzip the chromedriver.zip
5. Move the file to /usr/bin directory sudo mv chromedriver /usr/bin
6. Goto /usr/bin directory and you would need to run something like "chmod a+x chromedriver" to mark it executable.
7. finally you can execute the code.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://www.google.com")
display.stop()
Solution 3
For windows
Download webdriver from:
http://chromedriver.storage.googleapis.com/2.9/chromedriver_win32.zip
Paste the chromedriver.exe file in "C:\Python27\Scripts" Folder.
This should work now.
from selenium import webdriver
driver = webdriver.Chrome()
Solution 4
In addition to the selected answer (windows style path):
driver = webdriver.Chrome(executable_path=r"C:\Chrome\chromedriver.exe")
Note the r in front of the "C:\Chrome\chromedriver.exe", this makes this string a raw string.
In case you do not want to use a raw string you should escape the slash like so \\, this would become:
driver = webdriver.Chrome(executable_path="C:\\Chrome\\chromedriver.exe")
Or you can replace the \ with a /, you will get this:
driver = webdriver.Chrome(executable_path="C:/Chrome/chromedriver.exe")
Solution 5
For Debian/Ubuntu - it works:
install Google Chrome for Debian/Ubuntu:
sudo apt-get install libxss1 libappindicator1 libindicator7
wget https://dl.google.com/linux/direct/google-chrome-
stable_current_amd64.deb
sudo dpkg -i google-chrome*.deb
sudo apt-get install -f
Install ChromeDriver:
wget -N http://chromedriver.storage.googleapis.com/2.26/chromedriver_linux64.zip
unzip chromedriver_linux64.zip
chmod +x chromedriver
sudo mv -f chromedriver /usr/local/share/chromedriver
sudo ln -s /usr/local/share/chromedriver /usr/local/bin/chromedriver
sudo ln -s /usr/local/share/chromedriver /usr/bin/chromedriver
Install Selenium:
pip install -U selenium
Selenium in Python:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://www.google.co.in/')
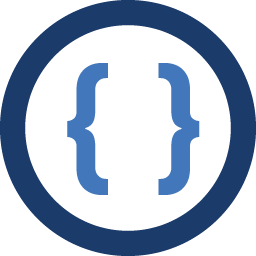
Admin
Updated on July 10, 2020Comments
-
Admin almost 4 years
I'm having trouble using the Chrome driver for Selenium. I have the chromedriver downloaded and saved to C:\Chrome:
driver = webdriver.Chrome(executable_path="C:/Chrome/")
Using that gives me the following error:
Traceback (most recent call last): File "C:\Python33\lib\subprocess.py", line 1105, in _execute_child startupinfo) PermissionError: [WinError 5] Access is denied During handling of the above exception, another exception occurred: Traceback (most recent call last): File "C:\Python33\lib\site-packages\selenium\webdriver\chrome\service.py", line 63, in start self.service_args, env=env, stdout=PIPE, stderr=PIPE) File "C:\Python33\lib\subprocess.py", line 817, in __init__ restore_signals, start_new_session) File "C:\Python33\lib\subprocess.py", line 1111, in _execute_child raise WindowsError(*e.args) PermissionError: [WinError 5] Access is denied During handling of the above exception, another exception occurred: Traceback (most recent call last): File "C:/Users/Wilson/Dropbox/xxx.py", line 71, in <module> driver = webdriver.Chrome(executable_path="C:/Chrome/") File "C:\Python33\lib\site-packages\selenium\webdriver\chrome\webdriver.py", line 59, in __init__ self.service.start() File "C:\Python33\lib\site-packages\selenium\webdriver\chrome\service.py", line 68, in start and read up at http://code.google.com/p/selenium/wiki/ChromeDriver") selenium.common.exceptions.WebDriverException: Message: 'ChromeDriver executable needs to be available in the path. Please download from http://chromedriver.storage.googleapis.com/index.html
Any help would be appreciated.
-
Marco Aurélio Deleu about 7 yearsWhy the
--no-sandbox
? -
Jaqueline Passos over 6 yearsWorked like a charm for Mac without the .exe estension
-
vikramvi about 6 yearsIsn't chromedriver should be put under /usr/local/bin directory in Ubuntu instead of /usr/bin ? please clarify
-
Jayakrishnan Nair about 6 yearsNote: Can use
apt
instead ofapt-get
in more recent versions of ubuntu. -
Corey Goldberg about 6 years@vikramvi it doesn't matter as long as the directory is on your path
-
vikramvi about 6 years@CoreyGoldberg I will prefer to put under default directory instead of custom directory
-
Tom over 4 yearsCan you add some further information as to how this helps solve the initial question?
-
Dave over 4 yearsWhile this code may solve the question, including an explanation of how and why this solves the problem would really help to improve the quality of your post, and probably result in more up-votes. Remember that you are answering the question for readers in the future, not just the person asking now. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply.
-
zero almost 4 yearshi there - whats the difference between
driver = webdriver.Chrome(executable_path="C:/Chrome/")
and this onedriver = webdriver.Chrome(executable_path=r"C:\Chrome\chromedriver.exe")
Note: i work on Win10 - i have issues with the chromedriver too - so i am working on the same things - look forward to hear from you -
zero almost 4 yearsgood evening dear Viky - many thanks for the great answer. BTW - one question left: hi there - whats the difference between
driver = webdriver.Chrome(executable_path="C:/Chrome/")
and this onedriver = webdriver.Chrome(executable_path=r"C:\Chrome\chromedriver.exe")
Note: i work on Win10 - i have issues with the chromedriver too - so i am working on the same things - look forward to hear from you -
falsetru almost 4 years@zero, Sorry, I don't have an access to windows 10 machine. Please post a separated question so that others with windows machine can answer you.
-
Victor Di over 3 yearsbefore installing latest version of chromium-browser make sure that you have the latest version of google chrome itself with >sudo apt install google-chrome-stable