Can I access ImageMagick API with Python?
Solution 1
I would recommend using Wand (explanations follows).
I was looking for proper binding to ImageMagick library, that would:
- work error/problem free
- be regularly maintained and up to date
- allow nice objective Python
But indeed python API (binding) has too many different (mostly discontinued) versions. After reading a nice historical overview by Benjamin Schweizer it has all become clear (also see his github wiki):
- GraphicsMagick
- PythonMagick - first implementation
- PythonMagickWand/Achim Domma - first Wand - a CDLL implementation
- PythonMagickWand/Ian Stevens
- MagickFoo - included in python-magickwand
- Wand/Hong Minhee - the latest project
Now Wand is just a (reduced) C API to the ImageMagick ".. API is the recommended interface between the C programming language and the ImageMagick image processing libraries. Unlike the MagickCore C API, MagickWand uses only a few opaque types. Accessors are available to set or get important wand properties." (See project homepage)
So it is already a simplified interface that is easer to maintain.
Solution 2
This has worked for me for the following command to create an image from text for the letter "P":
import subprocess
cmd = '/usr/local/bin/convert -size 30x40 xc:white -fill white -fill black -font Arial -pointsize 40 -gravity South -draw "text 0,0 \'P\'" /Users/fred/desktop/draw_text2.gif'
subprocess.call(cmd, shell=True)
Solution 3
I found no good Python binding for ImageMagick, so in order to use ImageMagick in Python program I had to use subprocess
module to redirect input/output.
For example, let's assume we need to convert PDF file into TIF:
path = "/path/to/some.pdf"
cmd = ["convert", "-monochrome", "-compress", "lzw", path, "tif:-"]
fconvert = subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = fconvert.communicate()
assert fconvert.returncode == 0, stderr
# now stdout is TIF image. let's load it with OpenCV
filebytes = numpy.asarray(bytearray(stdout), dtype=numpy.uint8)
image = cv2.imdecode(filebytes, cv2.IMREAD_GRAYSCALE)
Here I used tif:-
to tell ImageMagick's command-line utility that I want to get TIF image as stdout stream. In the similar way you may tell it to use stdin stream as input by specifying -
as input filename.
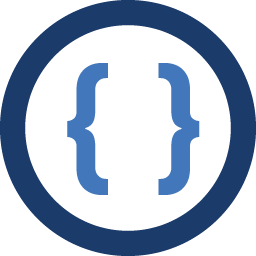
Admin
Updated on November 05, 2020Comments
-
Admin over 3 years
I need to use ImageMagick as PIL does not have the amount of image functionality available that I am looking for. However, I am wanting to use Python.
The python bindings (PythonMagick) have not been updated since 2009. The only thing I have been able to find is
os.system
calls to use the command line interface but this seems clunky.Is there any way to access the API directly using
ctypes
and conversions of some sort? As a last resort is there any other library out there that has the extensive amount of image editing tools like ImageMagick that I have looked over?