Can I disable the 'close' button of a form using C#?
Solution 1
You handle the Closing event (and not the Closed event) of the Form.
And then you use e.CloseReason to decide if you really want to block it (UserClose) or not (TaskManager Close).
Also, there is small example Disabling Close Button on Forms on codeproject.
Solution 2
Right click on the window in question, then click Properties. Under Properties click Events. Double click on the FormClosing
event.
The following code will be generated by Windows Form Designer:
private void myWindow_FormClosing(object sender, FormClosingEventArgs e)
{
}
Simply update the code to look like this (add e.Cancel = true;
):
private void myWindow_FormClosing(object sender, FormClosingEventArgs e)
{
e.Cancel = true;
}
You're done!
Alternatively, if you want to disable the close, maximize, and minimize buttons of the window:
You can right click the window in question, then click Properties. Under Properties set the ControlBox
property to false
.
Solution 3
If you are working with an MDI child window and disabling the close button during creation of the window is ruled out (i.e. you want to disable it at certain times during the form's life) none of the previously proposed solutions will work¹.
Instead we must use the following code:
[DllImport("user32")]
public static extern IntPtr GetSystemMenu(IntPtr hWnd, bool bRevert);
[DllImport("user32")]
public static extern bool EnableMenuItem(IntPtr hMenu, uint itemId, uint uEnable);
public static void DisableCloseButton(this Form form)
{
// The 1 parameter means to gray out. 0xF060 is SC_CLOSE.
EnableMenuItem(GetSystemMenu(form.Handle, false), 0xF060, 1);
}
public static void EnableCloseButton(this Form form)
{
// The zero parameter means to enable. 0xF060 is SC_CLOSE.
EnableMenuItem(GetSystemMenu(form.Handle, false), 0xF060, 0);
}
¹ You can set ControlBox = false
if you do not want any buttons, but that is not what the question is asking.
Solution 4
You should override the CreateParams
function derived from System.Windows.Forms.Form
And change the Class Style
myCp.ClassStyle = 0x200;
Solution 5
Closing += (s, eventArgs) =>
{
DialogResult = DialogResult.None; //means that dialog would continue running
};
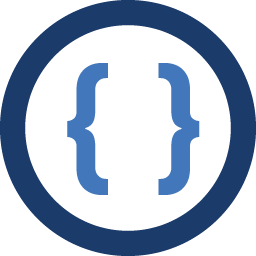
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
How can I disable the close button of a form like in the image below? (the image below show a
MessageBox
window)The
MessageBox
above was generated by me! I want to disable the close button of a normal form. -
Ric about 11 yearsThis is exactly what I was after! having minimal winform experience (previously asp.net only) this helped! thanks!