Can I set Eclipse to ignore "Unhandled exception type"
Solution 1
Is it possible to get Eclipse to ignore the error "Unhandled exception type FileNotFoundException".
No. That would be invalid Java, and Eclipse doesn't let you change the rules of the language. (You can sometimes try to run code which doesn't compile, but it's not going to do what you want. You'll find that UnresolvedCompilationError
is thrown when execution reaches the invalid code.)
Also note that just because the file existed when you called file.exists()
doesn't mean that it still exists when you try to open it a tiny bit later. It could have been deleted in the meantime.
What you could do is write your own method to open a file and throw an unchecked exception if the file doesn't exist (because you're so confident that it does):
public static FileInputStream openUnchecked(File file) {
try {
return new FileInputStream(file);
} catch (FileNotFoundException e) {
// Just wrap the exception in an unchecked one.
throw new RuntimeException(e);
}
}
Note that "unchecked" here doesn't mean "there's no checking" - it just means that the only exceptions thrown will be unchecked exceptions. If you'd find a different name more useful, then go for it :)
Solution 2
Declare that it throws Exception
Or put it in a try finally bolok
Solution 3
Here it is sir:
try
{
file = new File(filePath);
if(file.exists()) {
FileInputStream fileStream = openFileInput(filePath);
if (fileStream != null) {
// Do your stuff here
}
}
}
catch (FileNotFoundException e)
{
// Uncomment to display error
//e.printStackTrace();
}
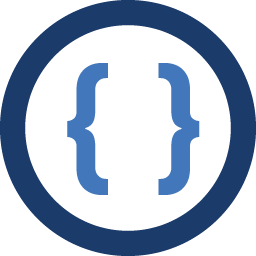
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
Is it possible to get Eclipse to ignore the error "Unhandled exception type"?
In my specific case, the reason being that I have already checked if the file exists. Thus I see no reason to put in a try catch statement.
file = new File(filePath); if(file.exists()) { FileInputStream fileStream = openFileInput(filePath); if (fileStream != null) {
Or am I missing something?
-
Admin about 10 yearsI understand the implications of ignoring exceptions. I come from a coding background that attempts to avoid try catch and handle these thing before they happen. I believe 'try catch' leads to lazy and inefficient code. The likelihood of the file been deleted between calls is highly unlikely its a private folder, meaning the only the app has access to the file. However I digress, If thats the way Java wants it done so be it...
-
Simon about 10 years@ChaosPixy I hear your sentiments but highly unlikely != impossible, especially in threaded applications, and try catch only leads to lazy inefficient code from lazy inefficient programmers! ;) The exceptions in my code, or at least I strive for, are only ever used if something happens outside my control. The ObjectiveC approach (which in my view, is as distasteful as the you see the Java approach) vs Java debate could rage for pages! PS. The downvote is silly, so I cancelled it out and then some.
-
Hassan almost 9 yearsA bit late to the party, but I wanted to say thanks. I come from a PHP/D background where unhandled exceptions automatically crash the program, but I couldn't find a way to replicate that behavior in Java. The syntax you suggested does just that.
-
Fratt about 6 yearsEven later to this party...but this seems like a clean answer, especially if you wish to handle thrown exceptions at the root of your call stack. So any class that you author and reference that throws an exception will bubble up and be handled in one place for maintainability. Marking your methods in the dependent class with "throws Exception" but handling them at the root of your call stack using this approach will work and not violate the language.