can SliverList be used inside TabBarView or vice versa?
1,084
So... I tried not to change your code too much :-). This Code works on Android:
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: MoboApp(),
);
}
}
class MoboApp extends StatefulWidget {
@override
_MoboAppState createState() => _MoboAppState();
}
class _MoboAppState extends State<MoboApp> with SingleTickerProviderStateMixin {
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 4,
child: Builder(builder: (BuildContext context) {
return NestedScrollView(
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
return <Widget>[
SliverAppBar(
leading: Icon(
Icons.list,
color: Color(0xFFFBF3F3),
),
actions: [
Icon(
Icons.more_vert,
color: Color(0xFFFBF3F3),
)
],
title: Text(
'mobo app',
style: TextStyle(
color: Color(0xFFFBF3F3),
fontSize: 25.0,
fontWeight: FontWeight.bold,
),
),
centerTitle: true,
pinned: true,
floating: false,
expandedHeight: 100.0,
bottom: TabBar(
unselectedLabelColor: Color(0xFFE0A1A1),
tabs: <Widget>[
Tab(
text: 'Home',
),
Tab(
text: 'Feed',
),
Tab(
text: 'Profile',
),
Tab(
text: 'Setting',
)
],
),
backgroundColor: Color(0xFFBB1A1A),
),
];
},
body: TabBarView(
children: [
// FIRST TabBarView
CustomScrollView(
slivers: [
SliverList(
delegate: SliverChildListDelegate(
[
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Image.network('https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg',
fit: BoxFit.cover,
),
),
),
],
),
SizedBox(
height: 10.0,
),
Row(
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Text('text'),
),
),
],
),
],
),
),
],
),
// SECOND TabBarView
CustomScrollView(
slivers: [
SliverList(
delegate: SliverChildListDelegate(
[
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Image.network('https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg',
fit: BoxFit.cover,
),
),
),
],
),
SizedBox(
height: 10.0,
),
Row(
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Text('text'),
),
),
],
),
],
),
),
],
),
//THIRD TabBarView
CustomScrollView(
slivers: [
SliverList(
delegate: SliverChildListDelegate(
[
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Image.network('https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg',
fit: BoxFit.cover,
),
),
),
],
),
SizedBox(
height: 10.0,
),
Row(
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Text('text'),
),
),
],
),
],
),
),
],
),
//FOURTH TabBarView
CustomScrollView(
slivers: [
SliverList(
delegate: SliverChildListDelegate(
[
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Image.network('https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg',
fit: BoxFit.cover,
),
),
),
],
),
SizedBox(
height: 10.0,
),
Row(
children: <Widget>[
Expanded(
child: Container(
color: Colors.white,
width: double.infinity,
child: Text('text'),
),
),
],
),
],
),
),
],
)
],
),
);
}),
);
}
}
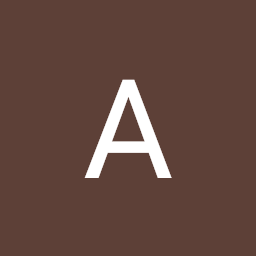
Author by
ch0or
Updated on December 29, 2022Comments
-
ch0or over 1 year
I am trying to used
SliverList
insideTabBarView
but I keep getting these errors:- Failed assertion: line 5966 pos 12: 'child == _child': is not true.
- A RenderRepaintBoundary expected a child of type RenderBox but received a child of type RenderSliverFillRemainingWithScrollable. Here is the code.
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: MoboApp(), ); } } class MoboApp extends StatefulWidget { @override _MoboAppState createState() => _MoboAppState(); } class _MoboAppState extends State<MoboApp> with SingleTickerProviderStateMixin { @override Widget build(BuildContext context) { return DefaultTabController( length: 4, child: Scaffold( body: CustomScrollView( slivers: <Widget>[ SliverAppBar( leading: Icon( Icons.list, color: Color(0xFFFBF3F3), ), actions: [ Icon( Icons.more_vert, color: Color(0xFFFBF3F3), ) ], title: Text( 'mobo app', style: TextStyle( color: Color(0xFFFBF3F3), fontSize: 25.0, fontWeight: FontWeight.bold, ), ), centerTitle: true, pinned: true, floating: false, expandedHeight: 100.0, bottom: TabBar( unselectedLabelColor: Color(0xFFE0A1A1), tabs: <Widget>[ Tab( text: 'Home', ), Tab( text: 'Feed', ), Tab( text: 'Profile', ), Tab( text: 'Setting', ) ], ), backgroundColor: Color(0xFFBB1A1A), ), TabBarView( children: [ // FIRST TabBarView SliverList( delegate: SliverChildListDelegate( [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Image.network( 'https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg', fit: BoxFit.cover, ), ), ), ], ), SizedBox( height: 10.0, ), Row( children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Text('text'), ), ), ], ), ], ), ), // SECOND TabBarView SliverList( delegate: SliverChildListDelegate( [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Image.network( 'https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg', fit: BoxFit.cover, ), ), ), ], ), SizedBox( height: 10.0, ), Row( children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Text('text'), ), ), ], ), ], ), ), //THIRD TabBarView SliverList( delegate: SliverChildListDelegate( [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Image.network( 'https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg', fit: BoxFit.cover, ), ), ), ], ), SizedBox( height: 10.0, ), Row( children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Text('text'), ), ), ], ), ], ), ), //FOURTH TabBarView SliverList( delegate: SliverChildListDelegate( [ Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Image.network( 'https://cdn.britannica.com/24/58624-050-73A7BF0B/valley-Atlas-Mountains-Morocco.jpg', fit: BoxFit.cover, ), ), ), ], ), SizedBox( height: 10.0, ), Row( children: <Widget>[ Expanded( child: Container( color: Colors.white, width: double.infinity, child: Text('text'), ), ), ], ), ], ), ), ], ) ], ), ), ); } }
-
Celt K. B. about 3 yearsYes, it is possible to use SliverList in a TabBarView. Could you add your code here?
-
ch0or about 3 years@CeltK.B. I have added my code. Please take a took at it i would really appreciate
-
ch0or about 3 yearsThank you so much. Much appreciated :) .
-
Celt K. B. about 3 yearsYou are welcome. You should accept the answer to close the question.
-
ch0or about 3 yearswill do that tanks