can somebody explain me what does "passing by value" and "Passing by reference" mean in C#?
Solution 1
In simple terms...
"Passing by value" means that you pass the actual value of the variable into the function. So, in your example, it would pass the value 9.
"Passing by reference" means that you pass the variable itself into the function (not just the value). So, in your example, it would pass an integer object with the value of 9.
This has various consequences, and each is useful in different situations.
This answer has more thorough information: What's the difference between passing by reference vs. passing by value?
Solution 2
Few Points:
In C# unless, you use ref keyword, it is always Pass By Value.
Pass By Value: In case of Value Type - It is just another copy of Value Type Variable.
In case of Reference Type - It is a copy of reference (or pointer) to exact location where memory is assigned in heap.
Pass By Reference:
for Reference type variable - passing it to another method by using keyword is only useful in the scenario when the passed variable can be reassigned in the called method. It means :
let's say - Outside (caller) method A inside (called) method B
Reference Type Object R -passed to method B from method A then if you reassign the memory location of Object R in method B then those changes wouldn't be seen in Method A of Object R unless you pass the object R using ref keyword (i.e pass by reference).
Solution 3
This is a very extensive subject so I will only try to answer your direct question. Passing something by value or reference to a method, will depend on what you're actually sending.
If it's a value type it will be sent by value, if it's a reference type it will send the reference by value. Basically it will send the variable itself to the method.
If you want true referencing, you have to use the ref
keyword.
An example to showcase it:
void Main()
{
int x = 5;
Console.WriteLine (x);
Test(x);
Console.WriteLine (x);
MyClass c = new MyClass();
c.Name = "Jay";
Console.WriteLine (c.Name);
TestClass(c);
Console.WriteLine (c.Name);
}
private void Test(int a){
a += 5;
}
private void TestClass(MyClass c){
c.Name = "Jeroen";
}
public class MyClass {
public String Name {get; set; }
}
Output:
5
5
Jay
Jeroen
An int
is a value type and thus its value is sent to the method. This means that any adjustment on that parameter will only be done on the local copy inside the method.
However, a class is a reference type and thus myClass
will send its memory location. This means that by changing the data inside the given object, this will also change the value of this class in different scopes because they both refer to the same memory location.
If you'd use the ref
keyword, the reference would be transmitted.
If you want more information, take it from a much more experienced person than me.
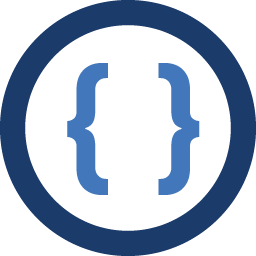
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am not quiet sure about the concept of "passing by value" and "passing by reference" in c#. I think Passing by value mean :
int i = 9;
we pass int i into a method like: method(i) Passing by reference means exactly passing its location like :
Class.method.variable
and it will give out the value. Can anybody help me out??
-
Lee over 10 yearsNeither of these are pass by reference. In C#, passing by reference requires using the
ref
orout
modifiers. -
crthompson over 10 years
In C# unless, you use ref keyword, it is always Pass By Value.
This is not correct. This is correct for simple types like int and bool, but a class is passed by reference by default. -
Lee over 10 years@paqogomez - Instances of class types are not passed by reference by default,
ref
orout
are required to pass by reference in C#. -
Jason Renaldo over 10 yearsAh, I thought he was asking just about the concept of passing reference or value types
-
Admin over 10 yearsThanks mate , this really helped ;)
-
newacct over 10 years@paqogomez: NO, it is 100% correct. Reference types work the same as all other types. Without
ref
orout
it is pass by value. -
newacct over 10 years"it will be sent by value by reference." what?
-
Jeroen Vannevel over 10 years@newacct: That did sound a little awkward.
-
Jason Renaldo over 10 years@Lee I'm curious, why does the selected answer address the same thing I do then?
-
Lee over 10 yearsAs it stands, your answer discusses the different between passing a reference type variable (
CustomObj
) by value, and passing a value type variable by value, namely that you can mutate an object through different copies of the same reference. The accepted answer explains that passing by reference creates an alias to the variable in the calling method, soobject o; SomeMethod(out o);
will actually causeo
itself to be modified, not just the object it is pointing to. -
GrandMasterFlush over 9 yearsJon Skeet clears this up quite well: stackoverflow.com/a/8708674/543538 and jonskeet.uk/csharp/parameters.html