Can static function access non-static variables in php?
Solution 1
No. A static method will not have access to $this
(as there is no $this
to talk about in a static context).
If you need a reference to the current object within the static method, it is not a static method. You can however access static properties and functions from a non-static method.
Solution 2
As the other answers say, you can't use the instance methods in a static method. However, you can store a static property being an array of your instances. With some code like this:
private static $_instances = array();
public function __construct() {
self::$_instances[] = $this;
}
This way, you can call a method on all the instances in a static method. With some code like this:
public static effItAll() {
foreach (self::$_instances as $instance) {
$instance->instanceMethod();
}
}
Or you could also just store the last instance. Or some instance depending on the parameters. Whatever, you can just store any instance in a static property, thus being able to then call this instance in your static method.
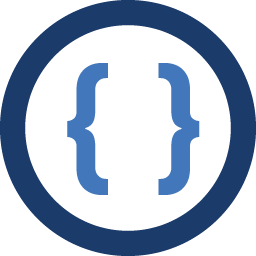
Admin
Updated on July 07, 2022Comments
-
Admin almost 2 years
<?php class Stat { public $var1='H'; public static $staticVar = 'Static var'; static function check() { echo $this->var1; echo "<br />".self::$staticVar ."<br />"; self::$staticVar = 'Changed Static'; echo self::$staticVar."<br />"; } function check2() { Stat::check(); echo $this->var1; echo "b"; } } ?>
Can i use it like this
$a = new Stat(); $a->check2();
-
Carlos Arturo Alaniz over 9 yearsYou could do a $instance = new self(); so that you could call the non static variables, it goes against the static element purpose but you could do it haha