Can You Switch PHP Sessions In a Session?
Solution 1
Here is a working example how to switch between sessions:
session_id('my1session');
session_start();
echo ini_get('session.name').'<br>';
echo '------------------------<br>';
$_SESSION['value'] = 'Hello world!';
echo session_id().'<br>';
echo $_SESSION['value'].'<br>';
session_write_close();
session_id('my2session');
session_start();
$_SESSION['value'] = 'Buy world!';
echo '------------------------<br>';
echo session_id().'<br>';
echo $_SESSION['value'].'<br>';
session_write_close();
session_id('my1session');
session_start();
echo '------------------------<br>';
echo $_SESSION['value'];
Log will look like:
PHPSESSID
------------------------
my1session
Hello world!
------------------------
my2session
Buy world!
------------------------
Hello world!
So, as you can see, session variables saved and restored while changing session.
Solution 2
Note: the answer below is not correct, please don't use or vote up. I've left it here as a place for discussion
You solution should work (not that I ever tried something like that), except that you have to manually close the previous session before any call to session_name()
as otherwise it will silently fail.
You can try something like this:
session_write_close();
$oldsession = session_name("MY_OTHER_APP_SESSION");
session_start();
$varIneed = $_SESSION['var-I-need'];
session_write_close();
session_name($oldsession);
session_start;
There's no need to actually mess with the session ID value, either through PHP session ID manipulation routines or through manual cookie mangling - PHP will take care of all that itself and you shouldn't mess with that.
Solution 3
I've been working on perfecting this and here is what I've come up with. I switch to a parent session using session names in my child apps and then back to my child app's session. The solution creates the parent session if it does not exist.
$current_session_id = session_id();
$current_session_name = session_name();
session_write_close();
$parent_session_name = 'NameOfParentSession';
// Does parent session exist?
if (isset($_COOKIE[$parent_session_name])) {
session_id($_COOKIE[$parent_session_name]);
session_name($parent_session_name);
session_start();
} else {
session_name($parent_session_name);
session_start();
$success = session_regenerate_id(true);
}
$parent_session_id = session_id();
// Do some stuff with the parent $_SESSION
// Switch back to app's session
session_write_close();
session_id($current_session_id);
session_name($current_session_name);
session_start();
Solution 4
The manual explains this pretty well but here's some example from the manual
session_start();
$old_sessionid = session_id();
session_regenerate_id();
$new_sessionid = session_id();
echo "Old Session: $old_sessionid<br />";
echo "New Session: $new_sessionid<br />";
print_r($_SESSION);
Related videos on Youtube
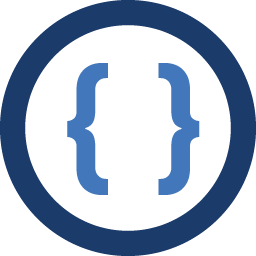
Admin
Updated on April 16, 2022Comments
-
Admin about 2 years
I have two apps that I'm trying to unify. One was written by me and another is a CMS I am using. My authentication happens in the one I coded and I'd like my CMS to know that information. The problem is that the CMS uses one session name, and my app uses another. I don't want to make them use the same one due to possible namespace conflicts but I'd still like to get this information.
Is it possible to switch session names in the middle of a request? For example, doing something like this in the CMS:
//session_start already called by cms by here $oldSession = session_name(); session_name("SESSION_NAME_OF_MY_APP"); session_start(); //get values needed session_name($oldSession); session_start();
Would something like this work? I can't find anything in the docs or on the web if something like this would work after session_start() has been called. Tips?
Baring this solution, I've been considering just developing a Web Service to get the information, but obviously just getting it from the session would be preferable as that information is already available.
Thanks!
-
hakre almost 13 years-1: This only changes the session id, but the session still is the same.
-
commonpike over 10 yearsIt seems to me, you can end up with two different session names that have the same id. If that happens, both sessions share the same data, even if they have different names. To avoid that, you may want to mess with the session id after all...
-
Guss over 10 yearsI don't see how that could happen - unless you assume that two different clients may also get the same session ID "by mistake". This is because the way session_name() works is that it changes what cookie PHP uses to identify the session, and if that causes you to generate a new session (instead of loading an existing one), PHP will guarantee that it is using a new session ID - otherwise it will be easy to hijack sessions.
-
Guss over 10 yearsAlso, messing around with the session ID is a sure way to open holes for session hijackers. If you do mess around with session IDs (please don't), don't assume you know how to generate session IDs and always use
session_regenerate_id()
. -
Guss over 10 yearsPlease don't mess with the session ID manually - this is what distinguishes one user from the other. If you manipulate session IDs by hand you allow session hijacking to occur, and that - for the uninitiated - is bad.
-
ajon over 10 yearsIn my tests this actually doesn't work. The second session_start() call will reopen the previously opened session... so whatever the session_id() was after the first session_start(). I have been searching and testing everywhere... you cannot switch sessions once you start. You can update the session id you can update the name, but the context and data will persist from your first session_start() call.
-
Guss over 10 yearsYou are correct - this doesn't actually work. I'm not sure if it ever worked (though I'm usually good about testing my answers... sorry). As far as I can tell,
session_start()
will always reload the previous session started, even if you change the session name. Changing the session name before the secondsession_start()
merely causes the creation of an additional session cookie with the same session ID (even if you usesession_regenerate_id()
before the secondsession_start()
). I did not find a way to not have the first session loaded, though I'm pretty sure this is a bug in PHP. -
Guss over 10 yearsInstead of manually setting session_id, you can call
session_regenerate_id()
(but you have to do it after the secondsession_start()
). Doing this change and removing bothsession_id()
calls from your code, you get the same effect without messing with session ids. Do note that this does not load an old session - all the data present in the "second session" is identical to the first session - it simply prevents the new data from being written to the old session. It does look like a start to build your own mechanism for managing exchangeable sessions. -
Roel van Duijnhoven almost 10 yearsThis worked for me! Changing back to the original session is important because otherwise magic session handling code at the end will be ran and things get weird!