Cancel a HTTP request
Solution 1
In Angular 2+, if you call unsubscribe on the request observable, the request will abort. The natural behaviour of subscription.unsubscribe() is not to abort the request, but angular 2 guys have added this specific functionality in angular/http package. Please refer this Angular github repo.
In normal scenarios (when not using angular/http), if you want to abort the request on unsubscribe, you may leverage below code -
Observable.create((observer) => {
function cancelRequest() {
// Clear any even listeners added previously.
request.abort();
}
// Other request processing related code
request.send(formData);
return () => {
cancelRequest();
};
});
Solution 2
Cancelling an HTTP request is a common requirement. For example, you could have a queue of requests where a new request supersedes a pending request and that pending request needs to be cancelled. Sign in to comment
To cancel a request we call the unsubscribe function of its subscription
@Component({ /* ... */ })
export class AppComponent {
/* ... */
search() {
const request = this.searchService.search(this.searchField.value)
.subscribe(
result => { this.result = result.artists.items; },
err => { this.errorMessage = err.message; },
() => { console.log('Completed'); }
);
request.unsubscribe();
}
}
Courtesy: Cancel http request
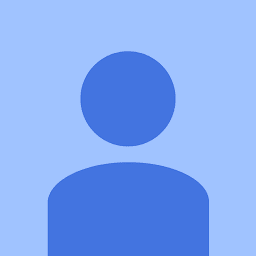
Daniel
Updated on June 04, 2022Comments
-
Daniel almost 2 years
I would like to know if there is any way I could check if a HTTP get is in pending status and if there is a way to cancel my HTTP get?
This is my HTTP get:
public getData(): Observable<any> { return this.httpClient.get('http://IP_ADRESS:3490/testStream', {responseType: 'arraybuffer', observe: 'response' }}); } public putData(): void { this.getData().subscribe((resp) => { console.log(resp); const responseArray: Uint8ClampedArray = new Uint8ClampedArray(resp); const newTabTemp: Uint8ClampedArray = new Uint8ClampedArray(this.maxHeight * this.maxWidth * 4); let comp = 0; for (let i = 0; i < this.maxHeight * this.maxWidth * 4; i = i + 4) { newTabTemp[i] = responseArray[comp]; newTabTemp[i + 1] = responseArray[comp]; newTabTemp[i + 2] = responseArray[comp]; newTabTemp[i + 3] = 255; comp = comp + 1; } let nouvData: ImageData; nouvData = new ImageData(newTabTemp, this.maxWidth, this.maxHeight); this.contextVideo.putImageData(nouvData, BORDERX / 2, BORDERY / 2); }); }
I'm getting a lot of these pending requests that never succeed or that take a long time to. How can I check if they're pending and cancel them?
-
alt255 over 5 yearsYou can unsubscribe from observable it will have the effect of cancellation
-