Cannot convert from type object to long
Solution 1
Try this:
Long a = (Long)itr.next();
You end up with a Long object but with autoboxing you may use it almost like a primitive long.
Another option is to use Generics:
Iterator<Long> itr = val.iterator();
Long a = itr.next();
Solution 2
Number
class can be used for to overcome numeric data-type casting.
In this case the following code might be used:
long a = ((Number)itr.next()).longValue();
I've prepared the examples below:
Object
to long
example - 1
// preparing the example variables
Long l = new Long("1416313200307");
Object o = l;
// Long casting from an object by using `Number` class
System.out.print(((Number) o).longValue() );
Console output would be:
1416313200307
Object
to double
example - 2
// preparing the example variables
double d = 0.11;
Object o = d;
// Double casting from an Object -that's a float number- by using `Number` class
System.out.print(((Number) o).doubleValue() + "\n");
Console output would be:
0.11
Object
to double
example - 3
Be careful about this simple mistake! If a float value is converted by using doubleValue()
function, the first value might not be equal to final value.
As shown below 0.11
!= 0.10999999940395355
.
// preparing the example variables
float f = 0.11f;
Object o = f;
// Double casting from an Object -that's a float number- by using `Number` class
System.out.print(((Number) o).doubleValue() + "\n");
Console output would be:
0.10999999940395355
Object
to float
example - 4
// preparing the example variables
double f = 0.11;
Object o = f;
// Double casting from an Object -that's a float number- by using `Number` class
System.out.print(((Number) o).floatValue() + "\n");
Console output would be:
0.11
Solution 3
Try : long a = ((Long) itr.next()).longValue();
Solution 4
You should use the new Generics features from Java 5.
When you take an element out of a Collection, you must cast it to the type of element that is stored in the collection. Besides being inconvenient, this is unsafe. The compiler does not check that your cast is the same as the collection's type, so the cast can fail at run time.
Generics provides a way for you to communicate the type of a collection to the compiler, so that it can be checked. Once the compiler knows the element type of the collection, the compiler can check that you have used the collection consistently and can insert the correct casts on values being taken out of the collection.
You can read this quick howto or this more complete tutorial.
Solution 5
in my case I have an array of Objects that I get from a flex client,
sometimes the numbers can be interpreted by java as int and sometimes as long,
so to resolve the issue i use the 'toString()' function as follows:
public Object run(Object... args) {
final long uid = Long.valueOf(args[0].toString());
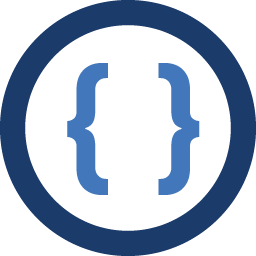
Admin
Updated on June 05, 2020Comments
-
Admin almost 4 years
I have a hashtable named
table
. The type value islong
. I am getting values using.values()
. Now I want to access these values.Collection val = table.values(); Iterator itr = val.iterator(); long a = (long)itr.next();
But when I try to get it, it gives me error because I can't convert from type
object
tolong
. How can I go around it? -
crazyscot about 14 yearsThis is more long-winded than it needs to be - see the other answer which refers to autoboxing.
-
missingfaktor about 14 years@crazyscot: From OP's code I assumed he's using an older version of Java than Java 5.