Cannot implicitly convert type 'System.Collections.Generic.IEnumerable<AnonymousType#1>' to 'System.Collections.Generic.List<string>
232,302
Solution 1
IEnumerable<string> e = (from char c in source
select new { Data = c.ToString() }).Select(t = > t.Data);
// or
IEnumerable<string> e = from char c in source
select c.ToString();
// or
IEnumerable<string> e = source.Select(c = > c.ToString());
Then you can call ToList()
:
List<string> l = (from char c in source
select new { Data = c.ToString() }).Select(t = > t.Data).ToList();
// or
List<string> l = (from char c in source
select c.ToString()).ToList();
// or
List<string> l = source.Select(c = > c.ToString()).ToList();
Solution 2
If you want it to be List<string>
, get rid of the anonymous type and add a .ToList()
call:
List<string> list = (from char c in source
select c.ToString()).ToList();
Solution 3
try
var lst= (from char c in source select c.ToString()).ToList();
Solution 4
If you have source as a string like "abcd"
and want to produce a list like this:
{ "a.a" },
{ "b.b" },
{ "c.c" },
{ "d.d" }
then call:
List<string> list = source.Select(c => String.Concat(c, ".", c)).ToList();
Solution 5
I think the answers are below
List<string> aa = (from char c in source
select c.ToString() ).ToList();
List<string> aa2 = (from char c1 in source
from char c2 in source
select string.Concat(c1, ".", c2)).ToList();
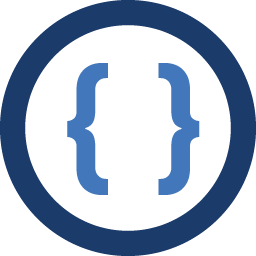
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have the code below:
List<string> aa = (from char c in source select new { Data = c.ToString() }).ToList();
But what about
List<string> aa = (from char c1 in source from char c2 in source select new { Data = string.Concat(c1, ".", c2)).ToList<string>();
While compile getting error
Cannot implicitly convert type
'System.Collections.Generic.List<AnonymousType#1>'
to'System.Collections.Generic.List<string>'
Need help.
-
Admin over 13 yearsI cannot use var.. it has to be List<string> because of some reason
-
abatishchev over 13 yearsThis way you will get
List<AnonymousType#1>
-
Coryza over 13 years@Rover: no it doesn't, .ToList() turns IEnumerable<AnonymousType#1> into List<AnonymousType#1>
-
Admin over 13 yearsWhat about List<string> aa = ( from char c1 in source from char c2 in source select new { Data = string.Concat(c1, ".", c2)).ToList<string>();
-
abatishchev over 13 years@priyanka.sarkar_2: You should use
Select(x => x.Data).ToList()
to select the list of such datas.