Cannot implicitly convert type 'System.DateTime?' to 'System.DateTime'. An explicit conversion exists
Solution 1
You have 3 options:
1) Get default value
dt = datetime??DateTime.Now;
it will assign DateTime.Now
(or any other value which you want) if datetime
is null
2) Check if datetime contains value and if not return empty string
if(!datetime.HasValue) return "";
dt = datetime.Value;
3) Change signature of method to
public string ConvertToPersianToShow(DateTime datetime)
It's all because DateTime?
means it's nullable DateTime
so before assigning it to DateTime
you need to check if it contains value and only then assign.
Solution 2
dt
is nullable
you need to access its Value
if (datetime.HasValue)
dt = datetime.Value;
It is important to remember that it can be NULL
. That is why the nullable
struct has the HasValue
property that tells you if it is NULL
or not.
You can also use the null-coalescing operator
??
to assign a default value
dt = datetime ?? DateTime.Now;
This will assign the value on the right if the value on the left is NULL
Solution 3
The problem is that you are passing a nullable type to a non-nullable type.
You can do any of the following solution:
A. Declare your dt
as nullable
DateTime? dt = dateTime;
B. Use Value
property of the the DateTime? datetime
DateTime dt = datetime.Value;
C. Cast it
DateTime dt = (DateTime) datetime;
Solution 4
you should be using the .Value of the datetime parameter. All Nullable structs have a value property which returns the concrete type of the object. but you must check to see if it is null beforehand otherwise you will get a runtime error.
i.e:
datetime.Value
but check to see if it has a value first!
if (datetime.HasValue)
{
// work with datetime.Value
}
Related videos on Youtube
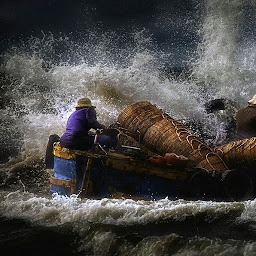
Amal K
21 year old Programming and Tech Enthusiast. Like exploring new languages and cultures. Currently pursuing my Bachelor's Degree. Working on my , and skills at the moment.
Updated on August 05, 2021Comments
-
Amal K almost 3 years
I am trying to convert
DateTime?
toDateTime
but I get this Error:Error 7 Cannot implicitly convert type 'System.DateTime?' to 'System.DateTime'. An explicit conversion exists
Here is my code:
public string ConvertToPersianToShow(DateTime? datetime) { DateTime dt; string date; dt = datetime; string year = Convert.ToString(persian_date.GetYear(dt)); string month = Convert.ToString(persian_date.GetMonth(dt)); string day = Convert.ToString(persian_date.GetDayOfMonth(dt)); if (month.Length == 1) { month = "0" + Convert.ToString(persian_date.GetMonth(dt)); } if (day.Length == 1) { day = "0" + Convert.ToString(persian_date.GetDayOfMonth(dt)); } //date = Convert.ToString(persian_date.GetYear(dt)) + "/" + Convert.ToString(persian_date.GetMonth(dt)) + "/" + //Convert.ToString(persian_date.GetDayOfMonth(dt)); date = year + "/" + month + "/" + day+"("+dt.Hour+":"+dt.Minute+")"; return date; }
-
Piotr Justyna over 10 yearsThis is your problem: dt = datetime; You should check if the datetime has value (HasValue) and then try to get it, or act accordingly if the value is not there.
-
-
Admin over 10 yearsI changed it some thing like that :DateTime dt=DateTime.Now;
-
Kamil Budziewski over 10 years@EhsanAkbar maybe change it to
DateTime dt = datetime??DateTime.Now
? -
David Pilkington over 10 yearsYes, as I mentioned in my answer. That way you get to keep your value if there is one
-
Millie Smith over 10 yearsI don't think ?? works here. Datetime on the left still can't be converted. I think you have to do datetime.HasValue ? datetime.Value : DateTime.Now
-
Kamil Budziewski over 10 years@MillieSmith nope,
??
means it, exactlydatetime.HasValue ? datetime.Value : DateTime.Now
it's just short version of that -
Millie Smith over 10 yearsI know that. I just don't remember the compiler figuring that out (even though it should be able to).
-
Cromwell over 8 years
??
works like a charm but we are using current date as the default. what is i want to assign, for example, 1.1.2000 as default date? -
Kamil Budziewski over 8 years@Cromwell use
dt = datetime??new DateTime(2000,1,1);