Cannot trigger google maps marker click event from html button
10,201
Here's corrected code...
<html>
<head>
<script src="http://maps.googleapis.com/maps/api/js?key=AIzaSyBl-XFi-PrFDZgDYRRD3PDtY4-xcRt6lkA&sensor=false">
</script>
</head>
<body>
<form method = "post">
<button name="semua" type="submit" value="">Semua</button>
<button name="makanan" type="submit" value="Ayam">Ayam</button>
</form>
<script>
var Coba1center = new google.maps.LatLng(-7.79793,110.36933);
var Coba1marker = new google.maps.Marker({
position:Coba1center,
});
var coba2center = new google.maps.LatLng(-7.78793,110.36933);
var coba2marker = new google.maps.Marker({
position:coba2center,
});
function initialize()
{
var mapCenter = new google.maps.LatLng(-7.79793, 110.36933);
var infowindow = null;
var mapProp = {
center:mapCenter,
zoom:12,
mapTypeId:google.maps.MapTypeId.ROADMAP
};
var map=new google.maps.Map(document.getElementById("googleMap")
,mapProp);
google.maps.event.addListener(Coba1marker,'click',function() {
map.setZoom(15);
map.setCenter(Coba1marker.getPosition());
if (infowindow) {
infowindow.close();
}
infowindow = new google.maps.InfoWindow();
infowindow.setContent("Coba1")
infowindow.open(map,Coba1marker);
});
Coba1marker.setMap(map);
google.maps.event.addListener(coba2marker,'click',function() {
map.setZoom(15);
map.setCenter(coba2marker.getPosition());
if (infowindow) {
infowindow.close();
}
infowindow = new google.maps.InfoWindow();
infowindow.setContent("itu")
infowindow.open(map,coba2marker);
});
coba2marker.setMap(map);
}
function showMarker(marker){
var gMarker = null;
var center = null;
console.log(marker);
if(marker === 'Coba1marker') {
gMarker = Coba1marker;
center = Coba1center;
}
else if(marker === 'coba2marker') {
gMarker = coba2marker;
center = coba2center;
}
console.log(gMarker);
console.log(center);
google.maps.event.trigger(gMarker, 'click', {
latLng: center
});
}
google.maps.event.addDomListener(window, 'load', initialize);
</script>
Coba1 <button type="button" onclick="showMarker('Coba1marker')">Click Me!</button> <br>
coba2 <button type="button" onclick="showMarker('coba2marker')">Click Me!</button> <br>
<div id="googleMap" style="width:500px;height:380px;"></div>
</body>
</html>
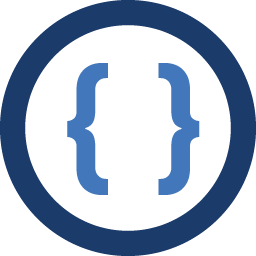
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I try to create a HTML button which can fire a click event on a marker in google maps, so whenever I click the button, the map will automatically zoom in to the marker and display the infowindow. Here's my code
<!DOCTYPE html> <html> <head> <script src="http://maps.googleapis.com/maps/api/js?key=AIzaSyBl-XFi-PrFDZgDYRRD3PDtY4-xcRt6lkA&sensor=false"> </script> </head> <body> <form method = "post"> <button name="semua" type="submit" value="">Semua</button> <button name="makanan" type="submit" value="Ayam">Ayam</button> </form> <script> function initialize() { var mapCenter = new google.maps.LatLng(-7.79793, 110.36933); var infowindow = null; var mapProp = { center:mapCenter, zoom:12, mapTypeId:google.maps.MapTypeId.ROADMAP }; var map=new google.maps.Map(document.getElementById("googleMap") ,mapProp); var Coba1center = new google.maps.LatLng(-7.79793,110.36933); var Coba1marker = new google.maps.Marker({ position:Coba1center, }); google.maps.event.addListener(Coba1marker,'click',function() { map.setZoom(15); map.setCenter(Coba1marker.getPosition()); if (infowindow) { infowindow.close(); } infowindow = new google.maps.InfoWindow(); infowindow.setContent("Coba1") infowindow.open(map,Coba1marker); }); Coba1marker.setMap(map); function showMarker(marker){ google.maps.event.trigger(marker, 'click'); } var coba2center = new google.maps.LatLng(-7.78793,110.36933); var coba2marker = new google.maps.Marker({ position:coba2center, }); google.maps.event.addListener(coba2marker,'click',function() { map.setZoom(15); map.setCenter(coba2marker.getPosition()); if (infowindow) { infowindow.close(); } infowindow = new google.maps.InfoWindow(); infowindow.setContent("itu") infowindow.open(map,coba2marker); }); coba2marker.setMap(map); } function showMarker(marker){ google.maps.event.trigger(marker, 'click'); } google.maps.event.addDomListener(window, 'load', initialize); </script> Coba1 <button type="button" onclick="showMarker(Coba1marker)">Click Me!</button> <br> coba2 <button type="button" onclick="showMarker(coba2marker)">Click Me!</button> <br> <div id="googleMap" style="width:500px;height:380px;"></div> </body> </html>
The map works almost perfectly; whenever I manually click the markers on the map, they behave as expected. However, when I click the buttons "Coba 1" and "coba2", they don't do anything. Also, any suggestions to make my code more elegant are welcomed (I'm not familiar with web programming).
EDIT Here's my working implementation with Jinja2:
<!DOCTYPE html> <html> <head> <script src="http://maps.googleapis.com/maps/api/js?key=AIzaSyBl-XFi-PrFDZgDYRRD3PDtY4-xcRt6lkA&sensor=false"> </script> </head> <body> <form method = "post"> <button name="semua" type="submit" value="">Semua</button> <button name="makanan" type="submit" value="Ayam">Ayam</button> </form> <script> {% for m in maps %} var {{ m.name }}{{ 'center' }} = new google.maps.LatLng({{m.latlon}}); var {{ m.name }}{{ 'marker' }} = new google.maps.Marker({ position:{{ m.name }}{{ 'center' }}, }); {% endfor %} function initialize() { var mapCenter = new google.maps.LatLng(-7.79793, 110.36933); var infowindow = null; var mapProp = { center:mapCenter, zoom:12, mapTypeId:google.maps.MapTypeId.ROADMAP }; var map=new google.maps.Map(document.getElementById("googleMap") ,mapProp); function setMarker(marker){ map.setZoom(15); map.setCenter(marker.getPosition()); if (infowindow) { infowindow.close(); } infowindow = new google.maps.InfoWindow(); } {% for m in maps %} google.maps.event.addListener({{ m.name }}{{ 'marker' }},'click',function() { setMarker({{ m.name }}{{ 'marker' }}); infowindow.setContent("{{ m.desc }}"); infowindow.open(map,{{ m.name }}{{ 'marker' }}); }); {{ m.name }}{{ 'marker' }}.setMap(map); {% endfor %} } function showMarker(marker){ google.maps.event.trigger(marker, 'click') } google.maps.event.addDomListener(window, 'load', initialize); </script> {% for m in maps %} {{ m.name }} <button type="button" onclick="showMarker({{ m.name }}{{ 'marker' }})">Click Me!</button> <br> {% endfor %} <div id="googleMap" style="width:500px;height:380px;"></div> </body> </html>