Cannot use a lambda expression as an argument to a dynamically dispatched operation
Solution 1
Your code works. The problem you are having isn't in the code you posted. This code runs.
void Main() {
int myObject = getValues("12").Sum(x => Int32.Parse(x.Price));
Console.WriteLine (myObject);
}
List<dynamic> getValues(string something) {
var item = new { Price = something };
IEnumerable<dynamic> items = Enumerable.Repeat<dynamic>(item, 2);
return items.ToList();
}
This produces the output 24
. The problem may be related to type inference, but that's just a guess. You should include enough code to reproduce the error for a more reliable answer.
Solution 2
As mentioned in the comment, this code is working fine for me:-
public static void Main()
{
var result = GetData("test").Sum(x => int.Parse(x.Name));
Console.WriteLine(result);
}
public static List<dynamic> GetData(string x)
{
List<dynamic> data = new List<dynamic>
{
new { Id =1, Name ="1"},
new { Id =2, Name ="4"},
new { Id =3, Name ="5"}
};
return data;
}
I am getting 10
as output.
Solution 3
so it ultimately turned out the issue was that I was passing a dynamic variable into the function call and subsequently using LINQ/lambda. Seems like that's a compiler no-no...
dynamic someVar = new {a=1,b=2};
int myObject = getValues(someVar.a).Sum(x => Int32.Parse(x.Price))
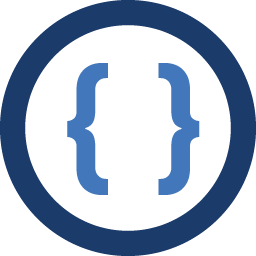
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to perform the following
int myObject = getValues(someVar).Sum(x => Int32.Parse(x.Price))
The function looks this:
List<dynamic> getValues(string something) {...}
This is the error I'm receiving: "Cannot use a lambda expression as an argument to a dynamically dispatched operation"
How can I SUM the values of a List object in a chained call similar to LINQ SUM?