Capturing image from webcam in java?
Solution 1
This JavaCV implementation works fine.
Code:
import org.bytedeco.javacv.*;
import org.bytedeco.opencv.opencv_core.IplImage;
import java.io.File;
import static org.bytedeco.opencv.global.opencv_core.cvFlip;
import static org.bytedeco.opencv.helper.opencv_imgcodecs.cvSaveImage;
public class Test implements Runnable {
final int INTERVAL = 100;///you may use interval
CanvasFrame canvas = new CanvasFrame("Web Cam");
public Test() {
canvas.setDefaultCloseOperation(javax.swing.JFrame.EXIT_ON_CLOSE);
}
public void run() {
new File("images").mkdir();
FrameGrabber grabber = new OpenCVFrameGrabber(0); // 1 for next camera
OpenCVFrameConverter.ToIplImage converter = new OpenCVFrameConverter.ToIplImage();
IplImage img;
int i = 0;
try {
grabber.start();
while (true) {
Frame frame = grabber.grab();
img = converter.convert(frame);
//the grabbed frame will be flipped, re-flip to make it right
cvFlip(img, img, 1);// l-r = 90_degrees_steps_anti_clockwise
//save
cvSaveImage("images" + File.separator + (i++) + "-aa.jpg", img);
canvas.showImage(converter.convert(img));
Thread.sleep(INTERVAL);
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Test gs = new Test();
Thread th = new Thread(gs);
th.start();
}
}
There is also post on configuration for JavaCV
You can modify the codes and be able to save the images in regular interval and do rest of the processing you want.
Solution 2
Some time ago I've created generic Java library which can be used to take pictures with a PC webcam. The API is very simple, not overfeatured, can work standalone, but also supports additional webcam drivers like OpenIMAJ, JMF, FMJ, LTI-CIVIL, etc, and some IP cameras.
Link to the project is https://github.com/sarxos/webcam-capture
Example code (take picture and save in test.jpg):
Webcam webcam = Webcam.getDefault();
webcam.open();
BufferedImage image = webcam.getImage();
ImageIO.write(image, "JPG", new File("test.jpg"));
It is also available in Maven Central Repository or as a separate ZIP which includes all required dependencies and 3rd party JARs.
Solution 3
JMyron is very simple for use. http://webcamxtra.sourceforge.net/
myron = new JMyron();
myron.start(imgw, imgh);
myron.update();
int[] img = myron.image();
Solution 4
This kind of goes off of gt_ebuddy's answer using JavaCV, but my video output is at a much higher quality then his answer. I've also added some other random improvements (such as closing down the program when ESC and CTRL+C are pressed, and making sure to close down the resources the program uses properly).
import java.awt.event.ActionEvent;
import java.awt.event.KeyEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.image.BufferedImage;
import javax.swing.AbstractAction;
import javax.swing.ActionMap;
import javax.swing.InputMap;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.KeyStroke;
import com.googlecode.javacv.CanvasFrame;
import com.googlecode.javacv.OpenCVFrameGrabber;
import com.googlecode.javacv.cpp.opencv_core.IplImage;
public class HighRes extends JComponent implements Runnable {
private static final long serialVersionUID = 1L;
private static CanvasFrame frame = new CanvasFrame("Web Cam");
private static boolean running = false;
private static int frameWidth = 800;
private static int frameHeight = 600;
private static OpenCVFrameGrabber grabber = new OpenCVFrameGrabber(0);
private static BufferedImage bufImg;
public HighRes()
{
// setup key bindings
ActionMap actionMap = frame.getRootPane().getActionMap();
InputMap inputMap = frame.getRootPane().getInputMap(JComponent.WHEN_IN_FOCUSED_WINDOW);
for (Keys direction : Keys.values())
{
actionMap.put(direction.getText(), new KeyBinding(direction.getText()));
inputMap.put(direction.getKeyStroke(), direction.getText());
}
frame.getRootPane().setActionMap(actionMap);
frame.getRootPane().setInputMap(JComponent.WHEN_IN_FOCUSED_WINDOW, inputMap);
// setup window listener for close action
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent e)
{
stop();
}
});
}
public static void main(String... args)
{
HighRes webcam = new HighRes();
webcam.start();
}
@Override
public void run()
{
try
{
grabber.setImageWidth(frameWidth);
grabber.setImageHeight(frameHeight);
grabber.start();
while (running)
{
final IplImage cvimg = grabber.grab();
if (cvimg != null)
{
// cvFlip(cvimg, cvimg, 1); // mirror
// show image on window
bufImg = cvimg.getBufferedImage();
frame.showImage(bufImg);
}
}
grabber.stop();
grabber.release();
frame.dispose();
}
catch (Exception e)
{
e.printStackTrace();
}
}
public void start()
{
new Thread(this).start();
running = true;
}
public void stop()
{
running = false;
}
private class KeyBinding extends AbstractAction {
private static final long serialVersionUID = 1L;
public KeyBinding(String text)
{
super(text);
putValue(ACTION_COMMAND_KEY, text);
}
@Override
public void actionPerformed(ActionEvent e)
{
String action = e.getActionCommand();
if (action.equals(Keys.ESCAPE.toString()) || action.equals(Keys.CTRLC.toString())) stop();
else System.out.println("Key Binding: " + action);
}
}
}
enum Keys
{
ESCAPE("Escape", KeyStroke.getKeyStroke(KeyEvent.VK_ESCAPE, 0)),
CTRLC("Control-C", KeyStroke.getKeyStroke(KeyEvent.VK_C, KeyEvent.CTRL_DOWN_MASK)),
UP("Up", KeyStroke.getKeyStroke(KeyEvent.VK_UP, 0)),
DOWN("Down", KeyStroke.getKeyStroke(KeyEvent.VK_DOWN, 0)),
LEFT("Left", KeyStroke.getKeyStroke(KeyEvent.VK_LEFT, 0)),
RIGHT("Right", KeyStroke.getKeyStroke(KeyEvent.VK_RIGHT, 0));
private String text;
private KeyStroke keyStroke;
Keys(String text, KeyStroke keyStroke)
{
this.text = text;
this.keyStroke = keyStroke;
}
public String getText()
{
return text;
}
public KeyStroke getKeyStroke()
{
return keyStroke;
}
@Override
public String toString()
{
return text;
}
}
Solution 5
Here is a similar question with some - yet unaccepted - answers. One of them mentions FMJ as a java alternative to JMF.
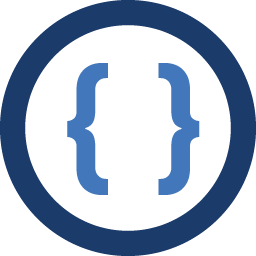
Admin
Updated on August 26, 2020Comments
-
Admin almost 4 years
How can I continuously capture images from a webcam?
I want to experiment with object recognition (by maybe using java media framework).
I was thinking of creating two threads
one thread:
- Node 1: capture live image
- Node 2: save image as "1.jpg"
- Node 3: wait 5 seconds
- Node 4: repeat...
other thread:
- Node 1: wait until image is captured
- Node 2: using the "1.jpg" get colors from every pixle
- Node 3: save data in arrays
- Node 4: repeat...
-
maple_shaft over 12 years+1 You rock! I was looking for a good open source and supported alternative to JMF for webcam captures in both Windows and Linux. This works like a charm! Thank you :)
-
Stepan Yakovenko almost 12 yearsDoesn't work for me, it says Exception in thread "main" java.lang.UnsatisfiedLinkError: C:\Users\steve\AppData\Local\Temp\javacpp213892357885346\jniopencv_core.dll: Can't find dependent libraries
-
syb0rg about 11 yearsThis will work, but the video quality isn't as good as it should be. See my answer for better video output quality.
-
mrswadge almost 11 yearsFor ease of getting started this is well worth a look. The code worked great for me, hopefully you also :)
-
Ilian Zapryanov over 10 yearsThank you for the suggestion. I am interested how to set it on Windows? Do I need the 3Gb extracted zip? Otherwise my program, using JavaCV on Linux works fine.
-
Java Man over 9 years@Bartosz Firyn : hello I am working with your API. awesome work man!but now I want to know that if zooming in camera supported or not? how to zoom camera using this API?
-
Bartosz Firyn over 9 years@JavaMan, there is no native support for zooming.
-
Java Man over 9 years@BartoszFiryn : okay thanks and what about drawing on camera? I want to draw Transparent frame on camera? then it is possible with API?
-
Bartosz Firyn over 9 years@JavaMan, you can draw either on the panel that display view from camera (WebcamPanel) by setting your custom Painter instance, or directly on the image that comes from camera by using WebcamImageTransformer feature. For more details please open the ticket on the Webcam Capture project page on Github since I do not want to explain this in details here on Stack Overflow (too much to write and too few characters to use in comments). The project can be found here github.com/sarxos/webcam-capture
-
Bartosz Firyn about 9 years@Elyas Hadizadeh, in case of problems you can always create new issue ticket at github.com/sarxos/webcam-capture where you can also find documentation and examples. Please also bear in mind that this is framework for people rather familiar with Java. In case you are Java newby there are also people willing to help, but you have to learn basics on your own.
-
Elyas Hadizadeh about 9 years@BartoszFiryn, I do agree with you, i must improve my knowledge, thank you for your reply. :-)
-
TaraW over 7 yearsThis is a great library. It covers all the needs for dynamic webcam picture capture. It even has motion detection capabilities. Great option.
-
fen1x almost 7 yearsYou should provide some explanation along with the code
-
Matthieu about 6 years@StepanYakovenko you need OpenCV installed.
-
Lucke over 5 yearsthanks. it's light and instead javacv this one works for me.
-
numX almost 4 years@BartoszFiryn is this library still actively maintained as there seem to be quite a few open issues and the last commit was quite a few months ago? Just asking to see if I should use this library or not, thanks!
-
Sandeep Malviya over 3 yearsI have used this but i got continues logs in console. How can i stop them like 2020-10-14 10:31:23.847 DEBUG 7108 --- [scovery-service] c.g.s.w.ds.buildin.WebcamDefaultDriver : Found device Integrated Webcam 0 2020-10-14 10:31:26.847 DEBUG 7108 --- [scovery-service] c.g.s.w.ds.buildin.WebcamDefaultDriver : Searching devices 2020-10-14 10:31:26.850 DEBUG 7108 --- [scovery-service] c.g.s.w.ds.buildin.WebcamDefaultDriver : Found device Integrated Webcam 0