Cardview with dividers in it
Solution 1
You can use this code this may help
<android.support.v7.widget.CardView
android:id="@+id/cardview"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="@dimen/margin_large"
android:layout_marginRight="@dimen/margin_large"
android:elevation="100dp"
card_view:cardBackgroundColor="@android:color/white"
card_view:cardCornerRadius="8dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:gravity="center_vertical"
android:paddingLeft="25dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Conversations" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="@android:color/darker_gray" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:paddingLeft="30dp"
android:paddingTop="20dp"
android:paddingBottom="10dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="game" />
...
</LinearLayout>
</LinearLayout>
</android.support.v7.widget.CardView>
Solution 2
The shown screenshot shows a normal CardView with views as divider in-between.
There is no DividerView or something similar if you searching for something like this. Just use a simple View
with a height and a background.
I've something similar in a library of mine. I use this to create the divider:
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@color/stroke"/>
card_library.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:paddingBottom="8dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="8dp"
android:layout_marginRight="8dp"
android:gravity="center_vertical"
android:orientation="horizontal">
<TextView
android:id="@+id/libraryname"
style="@style/CardTitle"
android:fontFamily="sans-serif-condensed"
android:textStyle="normal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:maxLines="1"/>
<TextView
android:id="@+id/libraryversion"
style="@style/CardTitle"
android:fontFamily="sans-serif-condensed"
android:textStyle="normal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="4dp"
android:layout_marginTop="4dp"
android:gravity="left|bottom"
android:maxLines="1"
android:textSize="12sp"/>
<TextView
android:id="@+id/librarycreator"
style="@style/CardTitle"
android:fontFamily="sans-serif-condensed"
android:textStyle="normal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="2dp"
android:gravity="right"
android:maxLines="2"
android:textSize="14sp"/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginTop="4dp"
android:background="@color/stroke"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_vertical"
android:padding="4dp">
<TextView
android:id="@+id/description"
style="@style/CardText"
android:fontFamily="sans-serif-condensed"
android:textStyle="normal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="8dp"
android:maxLines="20">
</TextView>
</LinearLayout>
</LinearLayout>
It will then look like this:
Solution 3
Set app:cardElevation="0dp" then use a View - see below
<android.support.v7.widget.CardView
android:id="@+id/cv"
xmlns:android="http://schemas.android.com/apk/res/android"
app:cardElevation="0dp"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
xmlns:app="http://schemas.android.com/apk/res-auto">
<!-- Insert UI elements -->
<View
android:layout_width="fill_parent"
android:background="@android:color/darker_gray"
android:layout_height="2dp"/>
</android.support.v7.widget.CardView>
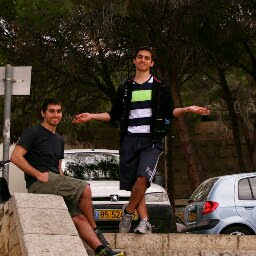
Amer Mograbi
Updated on July 09, 2022Comments
-
Amer Mograbi almost 2 years
I've seen those
CardViews
(I'm not sure they're evenCardViews
) with dividers used in a lot of apps, so I'm guessing there is an easy way to create them. I wanted to ask how is it exactly done? are those evenCardViews
?I couldn't find out more about them because I didn't know the name of the
View
exactly, so If someone could direct me to an example with code I'd be grateful.Image example: