Cast (const) char * to LPCWSTR
Solution 1
You can use the wide variants of cin and cout:
wchar_t input[256]; // don't really use a fixed size buffer!
wcout << L"Window title: ";
wcin >> input;
Solution 2
I would use:
#ifdef UNICODE
typedef std::wstring String;
#define Char wchar_t
#define out wcout
#define in wcin
#else
typedef std::string String;
#define Char char
#define out cout
#define in cin
#endif
String input;
out << _T("Window title: ");
in >> input;
FindWindow(input.c_str(), ...);
If you are sure if UNICODE
is defined you can do not make definition, but I recomend to use typedef for string type and use strings instead of api pointer types because it is type safer and it is easier to read code. In that case you need to call c_str()
method which returns pointer to the first element. Also you can use &string[0]
.
Solution 3
Why do you think you want LPCWSTR
? LPCWSTR
is used in programs compiled for UNICODE
. If you are using the char type everywhere then you should change the project property Character Set from 'Use UNICODE
character set' to 'Use Multibyte Character Set." If you do that then FindWindow
will accept a char array, like
char input[256];
Solution 4
First you could get users input as wchar_t*
instead of char*
. I think it would be the best option.
LPCWSTR
is a pointer to wide char array, so you need to convert every char
to wchar_t
.
So lets say you have:
char arr[] = "Some string";
So your actions:
size_t size = strlen(arr);
wchar_t* wArr = new wchar_t[size];
for (size_t i = 0; i < size; ++i)
wArr[i] = arr[i];
And if you need LPCWSTR
you just use &wArr[0]
(or some other index).
Important: don't forget to deallocate memory.
Related videos on Youtube
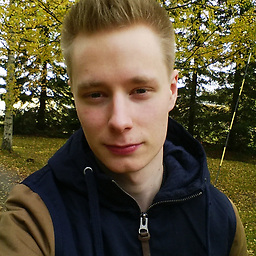
Markus Meskanen
Updated on September 14, 2022Comments
-
Markus Meskanen 3 months
I'm trying to use
FindWindow()
from WinAPI, and I want to ask an input for window's title from the user:char *input; cout << "Window title: "; cin >> input;
Pretty standard. Now then, how do I convert this to
LPCWSTR
forFindWindow()
?I've already tried the following:
_T(input)
,TEXT(input)
,(LPCWSTR)input
but none of them worked. I also tried usingwchar_t
instead ofchar
, but I needchar
everywhere else so then I get dozens of errors for usingwchar_t
instead ofchar
...-
David Heffernan over 9 yearsIf you are going to read in an ANSI string then you may as well call
FindWindowA
. If you want to support UTF-16, then you'd better stop storing strings in ANSI char arrays.
-
-
David Heffernan over 9 yearsWhy do you think you want LPCWSTR? Clearly because the compiler said so.