cast from [String:AnyObject] to unrelated type NSMutableDictionary always fails Warning
Solution 1
Try replacing
responseObject["data"].dictionaryObject! as! NSMutableDictionary
with this:
NSMutableDictionary(dictionary: responseObject["data"].dictionaryObject!)
You could easily cast it into a NSDictionary, but for some reason when you want a NSMutableDictionary, you have to initialize a new one with NSMutableDictionary(dictionary:)
Edit: see the comment on this question by @Tommy for why this is necessary.
Solution 2
Unlike NSArray
and NSDictionary
the mutable Foundation
collection types NSMutableArray
and NSMutableDictionary
are not bridged to the Swift counterparts.
The easiest solution is to keep using Swift native types
let parentView = self.parentViewController as! SBProfileViewController
parentView.savedDetailsModel = SBSavedUserModel(data:responseObject["data"].dictionaryObject!)
...
class SBSavedUserModel : NSObject {
var userId, firstName, lastName, imageBase64 : String
required init ( data : [String:AnyObject]) {
self.userId = data["userId"] as! String
self.firstName = data["fName"] as! String
self.lastName = data["lName"] as! String
self.imageBase64 = data["image"] as! String
}
}
Or – still more convenient if all values in the dictionary are strings
parentView.savedDetailsModel = SBSavedUserModel(data:responseObject["data"].dictionaryObject as! [String:String])
...
required init ( data : [String:String]) {
self.userId = data["userId"]!
self.firstName = data["fName"]!
self.lastName = data["lName"]!
self.imageBase64 = data["image"]!
}
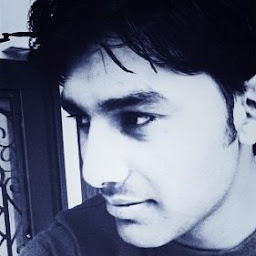
Jitendra
Passionate Swift and Objective C developer who loves his work. Passionate about technology, Software Development and learning new things every day.
Updated on June 11, 2022Comments
-
Jitendra almost 2 years
Code is working, but how do I silent this warning that keeps on appearing every time?
let parentView = self.parentViewController as! SBProfileViewController parentView.savedDetailsModel = SBSavedUserModel(data:responseObject["data"].dictionaryObject! as! NSMutableDictionary)
cast from '[String:AnyObject]' to unrelated type 'NSMutableDictionary' always fails Warning
SavedUserModel stores saved information:--
class SBSavedUserModel : NSObject { var userId : String! var firstName : String! var lastName : String! var imageBase64 : String! required init ( data : NSMutableDictionary) { self.userId = data.objectForKey("userId") as! String self.firstName = data.objectForKey("fName") as! String self.lastName = data.objectForKey("lName") as! String self.imageBase64 = data.objectForKey("image") as! String }
-
Tommy over 8 yearsThe "for some reason" is that you're starting with an immutable dictionary. It's not odd that the language won't allow you to try to mutate it. "This object is immutable" does not mean "this object requires slightly more arcane syntax to mutate".
-
Jitendra over 8 yearsSolution is correct however for some reasons I'm using NSMutableDictionary for mutable objects. Swift native types are always best choice if we are programming in swift only.