Cast with GetType()
Solution 1
Your intent is very unclear; however, one option is generics and MakeGenericMethod
in particular. What do you want to do with this? For example:
static class Program
{
static void Main()
{
object obj = 123.45;
typeof(Program).GetMethod("DoSomething")
.MakeGenericMethod(obj.GetType())
.Invoke(null, new object[] { obj });
}
public static void DoSomething<T>(T value)
{
T item = value; // well... now what?
}
}
So now we have the value, typed as double
via generics - but there still isn't much we can do with it except for calling other generic methods... what was it you want to do here?
Solution 2
I can't think of why you'd want to cast as GetType(), because you wouldn't be able to do anything to useful with the result, without knowing the type at compile time anyway.
Perhaps what you are looking for, is being able to Convert. If that is the case, the following should work for you:
object input = GetSomeInput();
object result = Convert.ChangeType(input, someOtherObject.GetType());
We use this when reading values from the registry which are all stored as strings, and then stuffing them into properties using reflection.
Solution 3
You can use the Activator.CreateInstance
method to create an instance from a type.
FYI reflection is SLOOWWWW, so if you need to do this cast many times in a row, it may be better to define your types in an enum or something like this then create instances without using reflection.
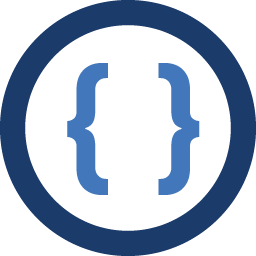
Admin
Updated on June 19, 2020Comments
-
Admin almost 4 years
Is it possible to cast an object to the type returned from
GetType()
? I'd like a generic method that can accept an object (for anonymous types) but then return an object cast as the anonymous type. I've been thinking of using the LCG DynamicMethod to build a method on a container class, but I can't exactly figure out what that would look like. The idea to cast with theGetType()
method was to be able to get the anonymous type and cast an object to its actual type without actually knowing the type.The overarching goal is to stick anonymous-typed objects into a container, that I could then share and pass between methods.