Change emoji Font for Flutter in Android
597
There is currently no way to define a custom font in Flutter just for emojis (or just for specified characters). There's an open Github issue about this.
There is however a trick to mix your font and another one for your emojis in a Text widget. I found an answer here to do that.
Here are the steps you can follow :
- You need to choose the emoji font you want to use. My favorite one is this Joypixels : https://www.joypixels.com/fonts
- Download the font .ttf and add it to your project, following the documentation : https://flutter.dev/docs/cookbook/design/fonts
- Add this code to your project :
class TextWithEmojis extends StatelessWidget {
const TextWithEmojis({
Key? key,
required this.text,
required this.fontSize,
required this.color,
}) : super(key: key);
final String text;
final double fontSize;
final Color color;
@override
Widget build(BuildContext context) {
return RichText(
text: _buildText(),
);
}
TextSpan _buildText() {
final children = <TextSpan>[];
final runes = text.runes;
for (int i = 0; i < runes.length; /* empty */) {
int current = runes.elementAt(i);
// we assume that everything that is not
// in Extended-ASCII set is an emoji...
final isEmoji = current > 255;
final shouldBreak = isEmoji ? (x) => x <= 255 : (x) => x > 255;
final chunk = <int>[];
while (!shouldBreak(current)) {
chunk.add(current);
if (++i >= runes.length) break;
current = runes.elementAt(i);
}
children.add(
TextSpan(
text: String.fromCharCodes(chunk),
style: TextStyle(
fontSize: fontSize,
color: color,
fontFamily: isEmoji ? 'Joypixels' : null,
),
),
);
}
return TextSpan(children: children);
}
}
- You can now use TextWithEmojis whenever you need :
TextWithEmojis(
text: "Hello 👋 😄",
fontSize: 12,
color: Colors.white
)
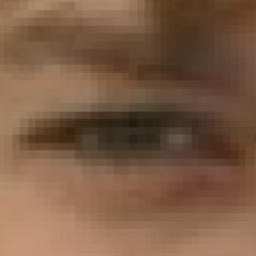
Author by
Nicolas Degen
Updated on December 29, 2022Comments
-
Nicolas Degen 5 months
Android has horrid emojis. Can I use Apple's emoji font (like Telegram does and WhatsApp used to) in Flutter for Android?
Edit: My first guess would be to add a font, but as I do not want to change all other fonts I am not sure if this is possible.
-
Jack' almost 2 yearshave you found a way yet ? I have the exact same issue.
-
-
Nicolas Degen about 2 yearsthat was not the question:) I was asking how to set the emoji font