Change "scroll to anchor" offset - jquery
Solution 1
It's probably because you are checking on the element's .scrollTop()
in the if
statement opposed to the actual body's scroll position. Because the $(this).scrollTop()
is probably static, the if statement won't make a difference.
So try:
if ($('body').scrollTop() > 1400) { ..
I've created an example in jsFiddle which demonstrates it works fine if you define the if
statement correctly:
> jsFiddle example <
If you scroll the body further down than 1400px, the scrolltop will scroll to:
$( $(this).attr('href') ).offset().top-165
(the color of the info div will also change color to green; makes it easy to test the setup)
Otherwise it will just scroll to:
$( $(this).attr('href') ).offset().top
That should help you out. Good luck.
Edit
If you only want the first anchor to have an offset, you can apply the following code:
// scroll to first anchor
$('a:first').click(function(){
$('body').animate({
scrollTop: $( $(this).attr('href') ).offset().top-165
}, 1400);
});
// scroll to other anchors
$('a:gt(0)').click(function() {
$('body').animate({
scrollTop: $( $(this).attr('href') ).offset().top
}, 1400);
});
This will give a scrollTop
offset of 165px only to the first anchor.
See the jsFiddle:
DEMO2
Solution 2
Thanks for the info! I'm doing it without Jquery and seems to work fine over diferent browsers (edge, firefox, chrome)
This is the simple html anchor:
<a class="anchorLink" name="rsc" id="rsc"></a>
And here the magic using negative margin top. Thats perfect when you have sticky headers.
.anchorLink {
float:left; width:100%; display:block; position:relative; margin-top:-163px;
}
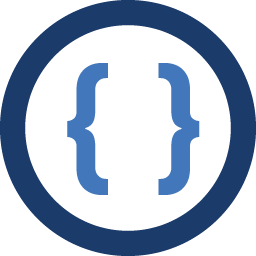
Admin
Updated on July 10, 2022Comments
-
Admin almost 2 years
Is it possible to change the offset of the scrollTo anchor jQuery?
At the first anchor I want a negative offset of 165px, but after that I don't want any offset.
My idea was something like the code below, but I can't make it work :/
Can anyone help me?
//scroll to anchor $('a').click(function(){ if ($(this).scrollTop() > 1400) { $('html, body').animate({ scrollTop: $( $(this).attr('href') ).offset().top-165 }, 1400); return false; } else { $('html, body').animate({ scrollTop: $( $(this).attr('href') ).offset().top }, 1400); return false; } });
-
Admin over 9 yearsThank you so much - That helped me on the right track! BUT now I have run into another problem.. When scrolling further than 1400px there is no longer the offset, so when I want to go back to the first anchor again the offset is missing. Is there anyway to overcome this? Maybe by setting the offset to only the first anchor somehow?
-
Jean-Paul over 9 years@kimkrog: Your intentions are not clear to me. If you look at my answer, you see that after 1400px the offset is removed by construction. Could you rephrase your question and construct a jsFiddle to show what you mean?
-
Admin over 9 yearsTry opening the jsFiddle that you posted. It workes fine when you press "click me" the first time, but if you then scroll to then bottom and press "click me" again it will go to the anchor, but with -165px offset. I want to to have the offset always when <= 1400px, also after you have passed 1400px the first time. Don't know if that made the question clearer, but let me know if not. And once again thank you for the help
-
Jean-Paul over 9 years@kimkrog: You do understand that this defeats the complete initial basis for your question? Why would there be an
if
statement for the 1400px if you don't want it to function? What you just described above would be this: jsFiddle. So no moreif
statement. Is that what you want??? -
Jean-Paul over 9 years@kimkrog: See the updated answer for the code where only the first
a
will have an offset of -165px and all the othera
will get no offset. -
Admin over 9 yearsThank you so much! The edited answer was exactly what I was looking for! Sorry that I couldn't be more clear in my question.. Thank you for taking the time to help me out :)
-
Jean-Paul over 9 years@kimkrog: No problem. Next time try preparing a jsFiddle to demonstrate what you are trying to do.