Change Spinner based on another Spinner Selection
String[] countries = {"INDIA","DUBAI","KSA"};
String[] country_code = {"91","971","966"};
final Spinner spinnerCountry = (Spinner)findViewById (R.id.spinnerCountry);
final Spinner spinnerCountryCode = (Spinner) findViewById (R.id.spinnerCountryCode);
ArrayAdapter<String> countryAdapter = new ArrayAdapter<String>(getApplicationContext(), android.R.layout.simple_list_item_1,countries);
ArrayAdapter<String> countryCodeAdapter = new ArrayAdapter<String>(getApplicationContext(), android.R.layout.simple_list_item_1,country_code);
spinnerCountry.setAdapter(countryAdapter);
spinnerCountryCode.setAdapter(countryCodeAdapter);
spinnerCountry.setOnItemSelectedListener(new OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> arg0, View arg1,int position, long arg3) {
spinnerCountryCode.setSelection(position);
}
@Override
public void onNothingSelected(AdapterView<?> arg0) {
}
});
spinnerCountryCode.setOnItemSelectedListener(new OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> arg0, View arg1,int position, long arg3) {
spinnerCountry.setSelection(position);
}
@Override
public void onNothingSelected(AdapterView<?> arg0) {
}
});
Here is some code that may fix your problem.
Explanation:
Assuming you have two spinners R.id.spinnerCountry and R.id.spinnerCountryCode.
As you can see i created two arrays which contains countryName and countryCode respectively.
Work Flow:-
When i select one item in a Spinner then the other spinner loads with another item corresponding to the position.
The code is Self-Explanatory!
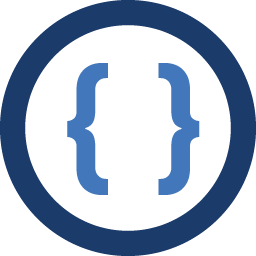
Admin
Updated on July 23, 2022Comments
-
Admin almost 2 years
I have string array which contains all the country names and another string array with respective country codes. I am setting the country names string to spinner. When I select the item from spinner its respective country code should be set to the spinner. And that item also should change to Code. Similarly if I select another item its code should be set to spinner and item too. But the first one I selected should change to its original country name. How should I do this?
XML
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.temp.MainActivity" > <Spinner android:id="@+id/spinnerCountry" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="124dp" /> </RelativeLayout>
Java Code
package com.example.temp; import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.widget.ArrayAdapter; import android.widget.Spinner; public class MainActivity extends Activity { final String[] countries = new String[] {"Afghanistan(AF)", "Albania(AX)", "Algeria(AL)", "American Samoa(DZ)", "Andorra(AS)"}; final String[] countrycodes = new String[]{"AF","AX","AL","DZ","AS"}; Spinner spinnerCountry; ArrayAdapter<String> arrayAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); spinnerCountry=(Spinner)findViewById(R.id.spinnerCountry); arrayAdapter = new ArrayAdapter<String>(getApplicationContext(), android.R.layout.simple_list_item_1,countries); spinnerCountry.setAdapter(arrayAdapter); } }
I can't share my original as I am using it in my application. But, I am sharing the same code like my original one. I want to set respective code when I select country from spinner.
-
erical about 8 yearsWhy do you use "final"?
-
theapache64 about 8 years@erical sometime i write code like that. that's all. :)
-
gautam almost 8 yearsfirst take an array for first spinner and according to your first array set value inside your json and after change in first spinner set the second one
-
Benjamin Vison about 7 years@erical because you are using the adapters inside the OnItemClickListeners you need to use final